在網頁開發中,靜態化頁面是一種將動態資料轉換為靜態HTML檔案的方法,這可以提高網站的效能與搜尋引擎優化(SEO)效果。以下是一個使用Idea、Servlet及FreeMarker來實現這個過程的案例指南。本指南假設您已經有基本的Java Web程式設計經驗並且瞭解如何使用Maven進行專案管理。
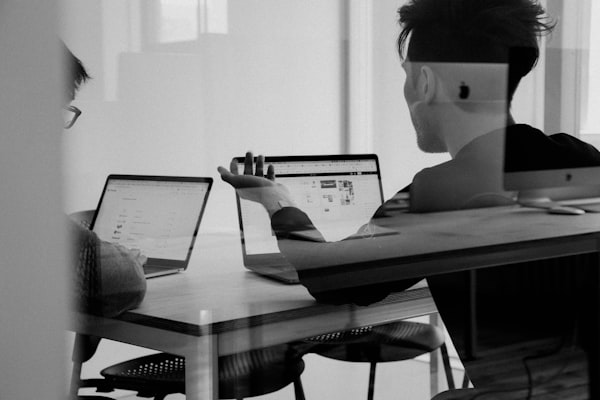
第1步:準備環境
首先,我們需要建立一個新的Maven專案並安裝必要的依賴項。打開您的IDE(例如IntelliJ IDEA或Eclipse),創建一個新專案,選擇“Maven”類型的專案。接著,配置POM.xml檔案以包含所需的依賴項。以下是一些常見的自由標記(FreeMarker)和相關的庫:
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.28</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
第2步:定義模型物件(Model Objects)
為了生成靜態頁面,我們需要定義用於表示資料的Java類別。這些類別通常稱為「模型物件」,它們會包含要在頁面上顯示的資料。例如,假設我們的網站有一個產品列表,我們可能會有如下的POJO(Plain Old Java Object):
public class Product {
private int id;
private String name;
// getters and setters omitted for brevity
}
第3步:編寫Servlet
下一步是撰寫一個Servlet,它可以根據使用者輸入的路徑來選取適當的模板文件(template file),並將其資料填入其中。這通常是透過FreeMarker模板語言完成的。以下是如何使用FreeMarker的簡要範例:
@WebServlet("/products/*")
public class ProductsServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String pathInfo = request.getPathInfo(); // e.g. /products/1 or /products/2
int productID = Integer.parseInt(pathInfo.substring(pathInfo.lastIndexOf('/') + 1));
Product product = getProductById(productID); // A method that fetches the product from database
Map<String, Object> model = new HashMap<>();
model.put("product", product); // Passing data to be displayed in template
// Prepare a FreeMarker template
Template template = Configuration.getDefaultConfiguration().getTemplate("productPage.ftlh");
// Generate HTML content using FreeMarker
Writer out = response.getWriter();
template.process(model, out);
out.close();
}
// Other methods like `getProductById` are not shown here as they depend on your specific application's data access layer
}
第4步:執行與測試
最後,您可以部署您的應用程式到伺服器上(例如Apache Tomcat)並開始測試。確保您的Servlet正確地獲取了資料並將其傳遞給了FreeMarker模板。在瀏覽器中訪問不同的URL路徑,查看生成的靜態HTML頁面是否符合預期。
總結來說,通過結合Idea作為IDE、Servlet API以及FreeMarker模板引擎,您可以輕鬆地實現從動態資料到靜態HTML頁面的轉換,從而提升網站的性能與SEO表現。隨著網站流量不斷增長,這種技術變得越來越重要,因為它可以減少對後端服務器的請求次數,從而降低負載。