在Spring Boot項目開發中,後端通常需要處理來自前端的參數傳遞。以下是幾種常見的方式來接收前端傳來的數據:
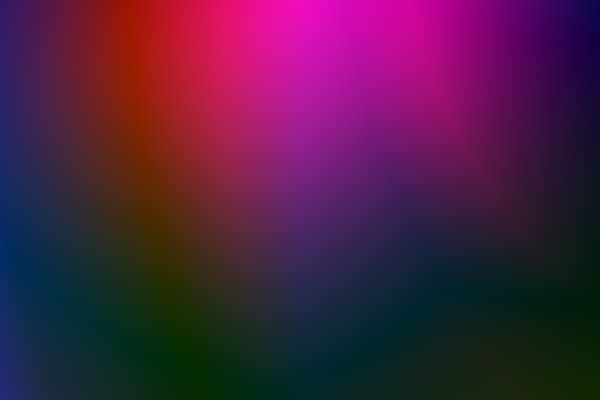
1. 傳統表單提交(Form Submission)
- 前端使用HTML form元素收集用戶輸入的數據,然後通過“的`method`屬性指定HTTP方法(通常是POST或GET),並通過`action`屬性指定指向後端控制器的路徑。
- 在後端控制器中,可以使用`@RequestParam`註解或者直接從`HttpServletRequest`對象獲取請求中的參數值。例如:
public class MyController {
@PostMapping("/saveUser") // POST方式提交
public void saveUser(@RequestParam String name, @RequestParam int age) {
// 根據name和age創建或更新用戶
}
}
2. JSON格式數據提交(JSON Body Parsing)
- 前端可以通過AJAX調用發送JSON格式的請求到後端。在後端,我們可以使用Jackson或其他庫將JSON字符串轉換爲Java對象。
- 以下是如何使用`@BodyParser`註解來解析JSON數據的示例:
import org.springframework.web.bind.annotation.*;
import com.fasterxml.jackson.databind.ObjectMapper;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@RestController
public class UserController {
private final ObjectMapper objectMapper = new ObjectMapper();
@PostMapping(value="/users", consumes=MediaType.APPLICATION_JSON_VALUE, produces={ MediaType.APPLICATION_JSON_VALUE })
public ResponseEntity createUser(@RequestBody User user, HttpServletResponse response) throws IOException {
response.setStatus(HttpServletResponse.SC_CREATED);
return ResponseEntity.created(new URI("api/v1/users/" + user.getId())).body(user);
}
public static class User {
int id;
String firstName;
String lastName;
LocalDate birthday;
// Getters and setters omitted for brevity
}
}
3. Multipart Form Data(文件上傳)
- 如果前端需要上傳文件,那麼會以multipart形式發送數據。在這種情況下,我們需要使用特定的工具類如`commons-fileupload`來處理上傳的文件。
- 以下是如何設置控制器接受multipart形式的例子:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.env.Environment;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartResolver;
import org.springframework.web.multipart.support.StandardServletMultipartResolver;
@Component
public class MultipartConfig implements org.springframework.context.ApplicationListener<org.springframework.context.event.ContextRefreshedEvent>{
private Environment env;
@Override
public void onApplicationEvent(ContextRefreshedEvent event) {
if (this.isMultipartRequired()) {
// Set multipart resolver
MultipartResolver multipartResolver = new StandardServletMultipartResolver(this.applicationContext.getBeanFactory());
AnnotationMethodHandlerAdapter adapter = (AnnotationMethodHandlerAdapter) this.applicationContext.getBean("&-----handlerAdapter", AnnotationMethodHandlerAdapter.class);
adapter.setOrder(Ordered.HIGHEST_PRECEDENCE);
adapter.setMessageConverters(this.getMessageConvertersWithMultiPartConfigured());
}
}
private boolean isMultipartRequired(){
return false; // 將false替換爲你項目的實際情況
}
private List<HttpMessageConverter<?>> getMessageConvertersWithMultiPartConfigured(){
List<HttpMessageConverter<?>> messageConverters = super.getMessageConverters();
messageConverters.add(0, new MultiPartHttpMessageConverter());
return messageConverters;
}
}
4. RESTful API接口
- Spring Boot支持構建RESTful APIs,其中經常涉及到複雜的對象序列化和解反序列化的操作。我們可以使用`@RequestBody`和`@ResponseBody`註解來處理這類情況。
- 下面是一個簡單的RESTful API樣例:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
@GetMapping("/greetings/{id}")
public Greeting greetingById(@PathVariable Long id) {
System.out.println("Got a request to retrieve the greeting with ID: " + id);
return new Greeting(id, "Hello, World!");
}
static class Greeting {
Long id;
String content;
Greeting(Long id, String content) {
this.id = id;
this.content = content;
}
}
}
5. WebSocket通信
- WebSocket提供了一種雙向實時通信機制,允許客戶端和服務端之間進行全雙工通信。在Spring Boot中,我們通常使用`@MessageMapping`和`@SendTo`註解來實現這個功能。
- 以下是一個簡化的WebSocket連接的例子:
@Configuration
public class WebSocketConfig extends AbstractWebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/wsendpoint").withSockJS();
}
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setClientInboundChannel(taskExecutor())
.setTaskQueueSize(16 * 1024)
.setThreadPoolMaxSize(32);
}
@Bean
public TaskScheduler taskScheduler() {
return new ConcurrentTaskScheduler();
}
@Bean
public Executor taskExecutor() {
return new SimpleAsyncTaskExecutor();
}
@Bean
public ApplicationRunner runner(final SimpMessagingTemplate template) {
return new ApplicationRunner() {
public void run(ApplicationArguments args, final ConfigurableApplicationContext context) throws Exception {
template.convertAndSend("/queue/hello", "Hello World");
}
};
}
}
以上只是Spring Boot中處理前端傳參的一些基本方法。在實際的項目中,你可能還會遇到更多複雜的情況,比如安全認證、國際化、異常處理等等。對於這些場景,你可以利用Spring Boot提供的豐富生態圈來解決相應的問題。