在開始之前,請確保您的計算機上安裝了Java開發套件(JDK)以及相應的編輯器或集成開發環境(IDE),如Eclipse或IntelliJ IDEA。這些工具將幫助您更輕鬆地創建、構建和運行基於Spring框架的應用程式。
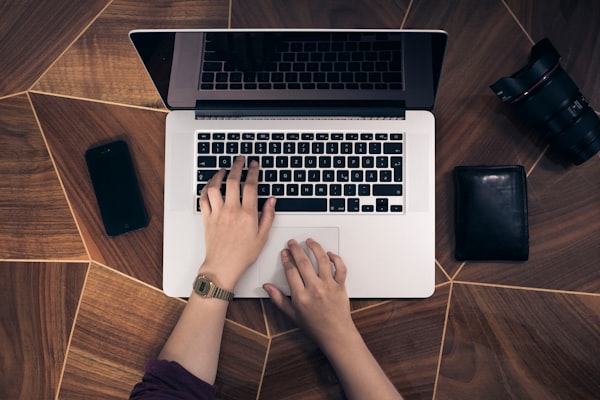
Spring的安裝與設置
1. 下載並解壓縮Spring框架
首先,從Spring官網或其他可靠來源下載最新版本的Spring框架。通常,Spring分為兩個主要部分:核心容器(Core Container)和數據訪問/融資支持(Data Access/Integration)。對於初學者來說,下載`spring-framework-5.x.RELEASE.zip`或更高版本即可。解壓縮後,您會得到一個包含多個jar檔案的目錄結構。
2. 配置Build Path或依賴性管理
如果您在使用Eclipse或IntelliJ IDEA,需要將Spring相關的jar檔案添加到專案的“Build Path”或“依賴性”(Dependencies)中。這將允許您在代碼中直接使用Spring框架中的類和介面。
3. 建立新的Maven專案(如果使用的話)
如果您正在使用Maven作為依賴性管理工具,那麼您可能需要在Maven中央庫中查找最新的Spring jar檔案,然後將它們添加到您的POM文件中。這樣可以讓Maven自動下載所需的Spring依賴項。
4. 引入Spring框架
在您的Java類中,您可以通過以下方式導入Spring的核心模塊:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
或者,如果您使用的是注釋驅動的方式,則需要引入:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
// 或者其他適用的註解,例如@Service, @Repository, @Controller
Spring的使用示例
這裡有一個簡單的例子,展示瞭如何在Spring中定義和使用bean:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 定義一個名為'greetingBean'的bean -->
<bean id="greetingBean" class="com.example.Greeting"/>
</beans>
在上述XML配置中,我們定義了一個名為`greetingBean`的對象,它的類型是`com.example.Greeting`。接下來,我們可以在我們的Java代碼中使用這個bean:
public class GreetingExample {
// 使用@Autowired註解來自動注入bean
@Autowired
private Greeting greetingBean; // 假設Greeting是Spring管理的bean
public void greet() {
System.out.println("Greetings from " + greetingBean.getMessage());
}
}
要啟用Spring的功能並獲得上述Java類中的 bean,您可以使用 `AnnotationConfigApplicationContext` 或 `ClassPathXmlApplicationContext`。以下是如何使用 XML 配置的範例:
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
GreetingExample example = (GreetingExample) context.getBean("greetingExample");
example.greet();
}
以上只是Spring框架的基本安裝和使用步驟。隨著經驗的積累,您將會學習更多高級特性,比如IoC(控制反轉)、AOP(面向切面的編程)、Spring Boot等等。記得查看官方文檔以獲取最詳細的信息。