在 Spring Boot 的 Web 開發中,Servlet 是不可或缺的一部分。Servlet(Server Applet)是一種服務器端 Java 程序,它被設計用來與 HTTP 協議進行交互以提供動態內容給用戶。在本文中,我們將探討如何在 Spring Boot 中使用 Servlet,以及如何通過不同的方式來註冊它們。
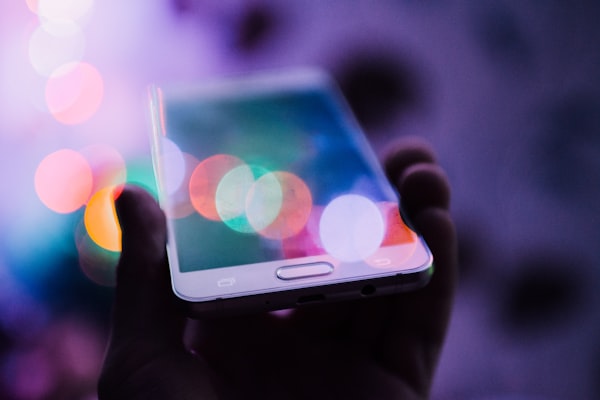
直接實現 `javax.servlet.Servlet` 接口
最基本的方式是通過繼承 `HttpServlet` 類或者直接實現 `javax.servlet.Servlet` 接口來實現一個 Servlet。以下是如何創建一個簡單的 Servlet 並在 Spring Boot 中註冊它的步驟:
1. 編寫 Servlet:創建一個新的 Java 類,繼承自 `HttpServlet` 或實現 `Servlet` 接口。在這個例子中,我們選擇繼承 `HttpServlet`:
public class HelloWorldServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.getWriter().println("Hello World from a Servlet!");
}
}
2. 配置 Servlet:在 Spring Boot 中,我們可以使用 `@WebServlet` 註解來配置 Servlet。這個註解可以放在 Servlet 類的上面,並且需要指定映射的 URL 和名稱:
@WebServlet(name = "helloWorldServlet", urlPatterns = "/hello-world")
public class HelloWorldServlet extends HttpServlet {
// 省略了具體的邏輯代碼
}
3. 註冊 Servlet:最後,我們需要確保 Servlet 在應用程序啓動時被加載。通常,這可以通過在 `applicationContext.xml` 文件中添加 XML 配置來實現,但在 Spring Boot 中,我們更傾向於使用 Java Configuration:
@Configuration
@EnableWebMvc // 如果使用了 Spring MVC
public class ServletConfig {
@Bean
public ServletRegistrationBean helloWorldServlet() {
return new ServletRegistrationBean(new HelloWorldServlet(), "/hello-world");
}
}
使用 `@Controller` 註解
如果你正在使用 Spring MVC,那麼你可以將 Servlet 視爲控制器(Controller)的一個特殊情況。在這種情況下,你可以像處理普通的 @Controller 一樣來處理 Servlet。這意味着你可以在方法上使用 `@RequestMapping` 註解來定義請求路徑,而無需顯式地註冊 Servlet。例如:
@Controller
public class MyServlet implements Serializable {
@RequestMapping(value="/myServlet", method=RequestMethod.GET)
public String handleRequest() {
return "Hello from my custom servlet!";
}
}
請注意,這種情況下,Servlet 需要實現 `Serializable` 接口,以便 Spring 將它標記爲單例模式。此外,由於它是基於 Spring MVC 的,因此需要在項目中引入相應的依賴項。
使用 `ServletContainerInitializer`
`ServletContainerInitializer` 提供了一種更加自動化的方式來註冊 Servlet。你需要做的就是提供一個實現了 `ServletContainerInitializer` 接口的 bean,並且在其中註冊所有的 Servlet。Spring Boot 會負責其餘的工作。以下是示例代碼:
import javax.servlet.ServletException;
import java.util.Set;
import javax.servlet.*;
public class CustomServletInitializer implements ServletContainerInitializer {
@Override
public void onStartup(Set<Class<?>> set, ServletContext servletContext) throws ServletException {
servletContext.addServlet(new ServletHolder(new HelloWorldServlet()), "/hello-world");
}
}
然後,在你的 Spring Bean 配置類中註冊這個 bean:
@Configuration
public class ApplicationConfig {
@Bean
public ServletContainerInitializer servletContainerInitializer() {
return new CustomServletInitializer();
}
}
使用 `@ServletComponentScan` 註解
如果在一個特定的包下有多個 Servlet,你可以使用 `@ServletComponentScan` 註解來自動掃描這些 Servlet 並將它們註冊到 Servlet Container 中。以下是在 Spring Boot 中使用此註解的方法:
1. 首先,確保你的 Servlets 位於要被掃描的包下:
package com.example.servlets;
public class AnotherServlet extends HttpServlet {}
2. 在你的 Spring Boot 主配置類中應用 `@ServletComponentScan` 註解:
@SpringBootApplication
@ServletComponentScan(basePackages = "com.example.servlets")
public class YourAppApplication {
// 省略其他配置
}
這樣,所有位於指定的 basePackage 中的 Servlet 都將被註冊。
無論哪種方式,都需要確保 Servlet 在 Spring Boot 上下文中可用,並且映射到正確的 URI 上。在實際的項目中,你可能需要根據自己的需求來選擇最適合的方案。