在本文中,我們將詳細介紹如何設定和使用 Vue 3 的富文編輯器 vue-quill-editor,以及圖片縮放功能 ImageResize。這兩個元件都是基於 Quill.js 開發的,Quill 是開源的富文編輯器 JavaScript 函式庫,提供強大的所見即所得 (WYSIWYG) 編輯體驗。
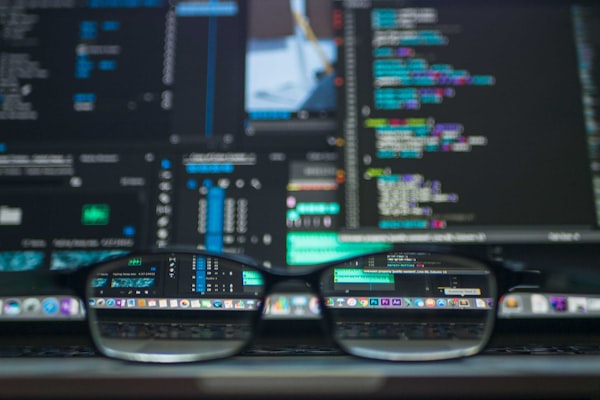
安裝與設定 vue-quill-editor
首先,您需要透過 npm 安裝 `vue-quill-editor` 和其依賴包 `quill`:
npm install quill vue-quill-editor --save
或者如果您使用 Yarn:
yarn add quill vue-quill-editor
接著,您需要在您的 Vue 專案中引入這些模組,並在入口檔案(如 main.js)中進行相應的設定:
import Vue from 'vue';
import App from './App.vue';
// Import the editor component and its dependencies
import { QuillEditor } from 'vue-quill-editor';
import 'quill/dist/quill.core.css'; // Core CSS file of Quill.
import 'quill/dist/quill.snow.css'; // Snow theme, if you want to use the snow theme.
import 'quill/dist/quill.bubble.css'; // Bubble theme, if you want to use the bubble theme.
Vue.component('QuillEditor', QuillEditor);
new Vue({
render: h => h(App),
}).$mount('#app');
使用 vue-quill-editor
現在您已經成功地將 `vue-quill-editor` 整合到您的 Vue 應用程式中了,您可以開始在你的 Vue 元件中使用它了。以下是如何使用的範例:
<!-- In your Vue component template -->
<template>
<div id="app">
<h1>Using vue-quill-editor</h1>
<QuillEditor ref="quillEditor" :modules="editorModules" />
</div>
</template>
<!-- In your Vue component script -->
<script>
export default {
data() {
return {
editorContent: '',
editorModules: {
toolbar: [
['bold', 'italic', 'underline'], // 文字格式化按鈕
[{ header: 1 }, { header: 2 }], // 大標題按鈕
[{'list': 'ordered'}, {'list': 'bullet'}], // 清單按鈕
[{'indent': '-1'}, {'indent': '+1'}], // 縮進按鈕
['link', 'image'], // 連結和圖片按鈕
['clean'] // 清除格式按鈕
],
clipboard: {
// Prevent removing image urls when pasting content
matchers: [
{
match: function(content, index, length) {
return /!\[(?:\[[^\]]*\])?\]\(([\s\S]*?)\)/.test(content);
},
run: function(content, index, length) {
const url = RegExp.$1;
return ['imagedata', { src: url }];
}
}
]
},
placeholder: '請輸入內容',
bounds: null,
readOnly: false
}
};
},
methods: {
getEditorHTML() {
console.log(`The HTML is: \n${this.$refs.quillEditor.quill.root.innerHTML}`);
},
setEditorText('Hello World!') {
this.$nextTick(() => {
this.editorContent = this.$refs.quillEditor.quill.setText();
});
}
}
};
</script>
在上面的範例中,`editorModules` 定義了工具列上的可用選項,並且包含了圖片的支援。`clipboard` 中的 `matchers` 用來處理當用戶從剪貼簿粘貼內容時,防止圖片 URL 被移除。`placeholder` 則是為空白的編輯區域顯示一個提示訊息。
使用 ImageResizer
ImageResizer 是一個額外的插件,它可以讓使用者調整插入的圖片的大小。首先,您需要安裝這個插件:
npm i @quill/image-resize-module@latest
# or using yarn:
yarn add @quill/image-resize-module@latest
然後,在您的 `editorModules` 中加入對它的支援:
editorModules: {
// ... other modules
formats: [
// Add support for resizing images
'block', 'inline', 'size', 'color', 'background', 'font', 'align',
'header', 'list', 'bullet', 'indent', 'direction', 'link', 'video',
'addition', 'subtraction', 'resizemode', 'float', 'spacing', 'blockquote'
],
// Enable modules that will be used in toolbar buttons
toolbar: [
// Resize module must come after inline
['bold', 'italic', 'underline', 'strike'],
[{ 'header': 1 }, { 'header': 2 }, { 'header': 3 }],
[{ 'list': 'ordered' }, { 'list': 'bullet' }, { 'indent': '-1' }, { 'indent': '+1' }],
[{ 'direction': 'rtl' }],
[{ 'size': ['small', false, 'large', 'huge'] }],
[{ 'color': [] }, { 'background': [] }],
[{ 'font': [] }],
[{ 'align': [] }],
['clean'],
['link', 'image'],
['smiley', 'code-block'],
['fullscreen', 'media', 'inserttable', 'draftmode'],
// Add image resize handler at bottom
['imageresize', { size: [null, '50%', '100%'] }]
],
// ... other options
}
注意,您必須確保 `imageresize` 在工具列的最後面出現,因為它是作為一個 “操作” 而不是一個格式化命令。此外,`size` 選項允許使用者選擇圖片的尺寸比例。
總結
在本文中,我們探討瞭如何在 Vue 3 中設定和使用 `vue-quill-editor` 以及如何添加 `ImageResize` 功能。這些工具提供了靈活的解決方案,幫助開發人員輕鬆地在網頁應用程式中實現豐富的內容編輯體驗。通過合理的設定和適當的使用,可以有效地提高產品的生產力和滿意度。