在現代企業級開發中,Spring Boot 因其快速開發、低學習成本和高生產力的特點而廣受歡迎。MyBatis Plus(簡稱 MP)則是一款優秀的持久層框架,它基於 MyBatis 進行了增強封裝,提供了更加簡潔的 API 和一系列提高開發效率的功能。本文將介紹如何使用 Spring Boot 整合 MyBatis Plus Generator (MPG) 進行數據庫表結構映射生成對應的實體類、Mapper.xml 和領域模型代碼。
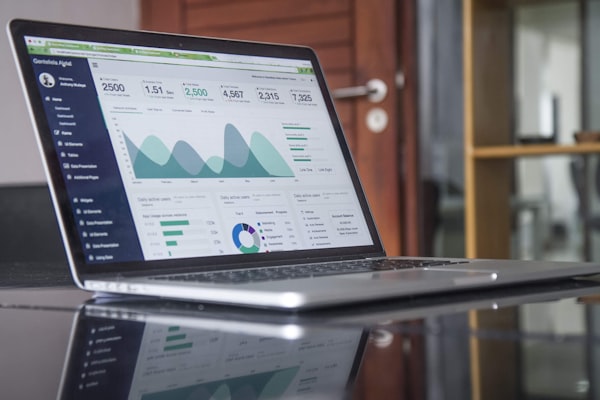
準備工作
1. 環境搭建:確保你已經安裝了 JDK、Maven 等基礎軟件,並且熟悉 Spring Boot 的基本配置和使用方法。
2. 項目創建:通過 IntelliJ IDEA 或 Eclipse 等集成開發環境新建一個 Spring Boot 項目,或者直接從 Maven Central Repository 中導入相關的依賴項。
3. 依賴管理:在你的項目的 `pom.xml` 文件中添加以下必要的依賴項:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本號</version>
</dependency>
<!-- 如果需要使用 MPG,還需要引入這個依賴 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>最新版本號</version>
</dependency>
4. 配置文件:在你的應用配置文件中添加相關配置,例如:
# mybatis-plus配置
mybatis-plus:
mapper-locations: classpath*:**/*.xml
typeAliasesPackage: com.example.project.entity
global-config:
db-config:
table-underline: true # 表名是否帶有下劃線風格
logic-delete-value: -1 # 邏輯已刪除值(默認爲-1)
logic-not-delete-value: 0 # 邏輯未刪除值(默認爲0)
5. 數據庫準備:確保你有權限訪問目標數據庫,並且在其中已經存在你要處理的數據表。
集成 MyBatis Plus Generator
1. MPG插件配置:在你的項目中添加 MPG 的配置文件,通常命名爲 `mybatis-plus-generator.xml`,內容如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN"
"http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd">
<generatorConfiguration>
<!-- 定義要掃描的包,用於自動識別 model 中的 domainObject -->
<package name="com.example.project.model"/>
<!-- 自定義全局策略 -->
<context id="baseModelContext" targetRuntime="MyBatis3">
<!-- 開啓駝峯命名轉換, -->
<plugin type="org.mybatis.generator.plugins.RenameToCamelCasePlugin" />
<!-- 禁用掉其它的模板, 僅保留字段複製到屬性 -->
<plugin type="org.mybatis.generator.internal.DefaultShellGeneratorPlugin">
<property name="skipAllQueries" value="true" />
</plugin>
</context>
<!-- 指定生成的文件保存路徑 -->
<javaTypeResolver>
<property name="forceBigDecimals" value="false" />
</javaTypeResolver>
<!-- 實體類的作者信息 -->
<commentGenerator>
<property name="author" value="Your Name Here"/>
</commentGenerator>
<!-- 輸出文件編碼格式 -->
<configuration options="java8Mode">
<property name="characterEncoding" value="utf-8"/>
</configuration>
</generatorConfiguration>
2. 運行 MPG 任務:在 Spring Boot 應用程序啓動後,你可以通過調用特定的接口來觸發 MPG 生成相應的代碼。下面是一個簡單的示例控制器實現:
@RestController
public class MpgController {
@Autowired
private MapperFactory mapperFactory;
/**
* 執行MPG生成器
*/
@GetMapping("/mpg")
public String generate() {
List<String> warnings = new ArrayList<>();
File directory = new File("src/main/resources/mapping"); // 設置生成文件的目錄
if (directory.exists()) {
System.out.println("[清理舊數據]");
FileUtil.del(directory);
} else {
System.out.println("[創建新文件夾]");
directory.mkdirs();
}
try {
mapperFactory.initGlobalConfig();
List<Class<?>> entityClasses = new ArrayList<>(Arrays.asList(this.getClass().getClassLoader().loadClasses("com.example.project.domainobject")));
Map<Class<?>, List<TableInfo>> tableInfoList = mapperFactory.getEntityMetaData(warnings, entityClasses);
for (Entry<Class<?>, List<TableInfo>> entry : tableInfoList.entrySet()) {
new MappingBuilder(entry.getValue(), directory).build();
}
return "success";
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("生成失敗" + warnings);
}
}
}
3. 測試與驗證:通過調用上述的 HTTP API 來觸發 MPG 生成代碼,然後檢查生成的文件是否符合預期。如果一切正常,你應該會在指定的目錄中發現新的實體類、Mapper.xml 和領域模型的 Java 源碼。
最佳實踐
1. 定期更新: 根據數據庫結構的變更定期運行 MPG 任務以確保你的代碼始終是最新的。
2. 自動化構建: 將 MPG 作爲持續集成的一部分,這樣每次提交代碼都會觸發一次代碼生成。
3. 模塊化設計: 將領域對象、映射器和 SQL 語句分開存儲在不同模塊中以便於維護和管理。
4. 安全性考慮: 在實際環境中,你可能需要在 MPG 任務的執行上增加額外的安全措施以防止惡意操作。
通過將 Spring Boot 與 MyBatis Plus Generator 相結合,我們可以顯著提升數據庫表結構映射到領域對象的自動化程度。這不僅節省了手動編寫大量重複性代碼的時間,還提高了整個團隊的開發效率。在實際工作中,可以根據具體需求對 MPG 進行定製化配置,從而獲得更好的開發體驗。