在Java後端開發中,當需要將對象序列化爲JSON格式的數據返回給前端時,如果某個屬性的值是`null`或者想要忽略某些特定的屬性,我們可以通過使用Jackson庫中的`ObjectMapper`來實現這個功能。以下是如何實現的方法說明:
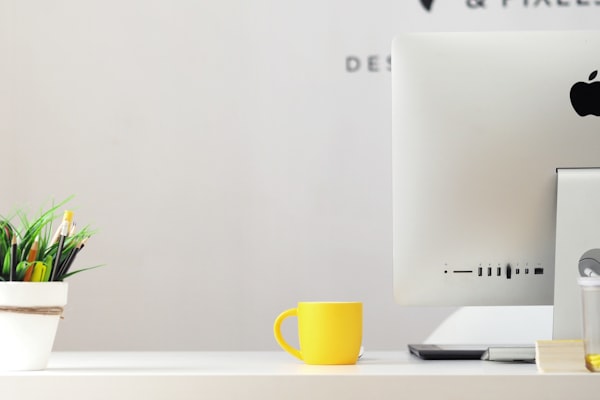
1. 創建一個簡單的Java類
首先,我們需要定義一個包含多個屬性的Java類來模擬我們的模型對象。例如:
public class Person {
private int id;
private String name;
private Integer age; // 注意這裏使用了Integer而不是int類型
private Address address;
}
class Address {
private String city;
// 其他字段省略...
}
2. 配置Jackson ObjectMapper
在使用`ObjectMapper`進行序列化之前,我們可以對其進行一些配置以達到我們所需的效果:
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.datatype.jdk8.JDK8Module;
import com.fasterxml.jackson.module.jaxb.JaxbAnnotationModule;
public class JacksonConfig {
public static final ObjectMapper MAPPER = new ObjectMapper()
.registerModule(new JAXBAdapterModule())
.enable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS)
.disable(SerializationFeature.FAIL_ON_EMPTY_BEANS);
}
在上述代碼中,我們註冊了一個模塊以便於序列化時轉換爲XML兼容的日期格式(如果你不需要這種格式,可以不註冊),並且禁用了`SerializationFeature.FAIL_ON_EMPTY_BEANS`特性,這樣即使對象中有`null`值的屬性也不會導致序列化失敗。
3. 過濾掉值爲空的屬性
要過濾掉值爲空的屬性,我們可以使用`filterValueS()`方法:
Person person = new Person();
person.setId(100);
person.setName("John Doe");
person.setAge(null); // Age is null, we want to filter it out
Address address = new Address();
address.setCity("New York City");
person.setAddress(address); // We also want to ignore the `address` field
String json = JacksonConfig.MAPPER.writerWithDefaultPrettyPrinter()
.writeValueAsString(person);
System.out.println(json);
上述代碼將會輸出如下結果:
{
"id": 100,
"name": "<NAME>"
}
你可以看到,`age`屬性和`address`屬性都被忽略了,因爲它們的值分別是`null`或者沒有設置值。
4. 忽略指定的屬性
如果我們只想忽略某些特定屬性而不考慮其值是否爲`null`,可以使用`excludeFieldsWithoutExposeAnnotation()`方法:
Person person = new Person();
person.setId(100);
person.setName("John Doe");
person.setAge(null);
Address address = new Address();
person.setAddress(address);
String json = JacksonConfig.MAPPER.writerWithDefaultPrettyPrinter()
.writeValueAsString(person);
System.out.println(json);
在這個例子中,`age`屬性會被忽略,因爲它沒有被明確地設置爲應該被序列化的狀態,而`address`屬性則不會被忽略。
5. 實際應用
在實際項目中,你可能需要在API層面上提供更細粒度的控制來決定哪些屬性應該被序列化以及哪些不應該被序列化。這可以通過在前端發送請求時指定所需的字段來完成,也可以在後端提供不同的接口來進行不同程度的暴露。無論哪種方式,理解如何使用Jackson來進行定製化的序列化是非常重要的。