在Spring MVC框架中,`org.springframework.web.bind.MissingServletRequestParameterException` 是一種常見的異常類型,它表示請求中缺少了控制器(Controller)所期望的某個HTTP參數或查詢字符串參數。這個異常通常發生在以下兩種情況下:
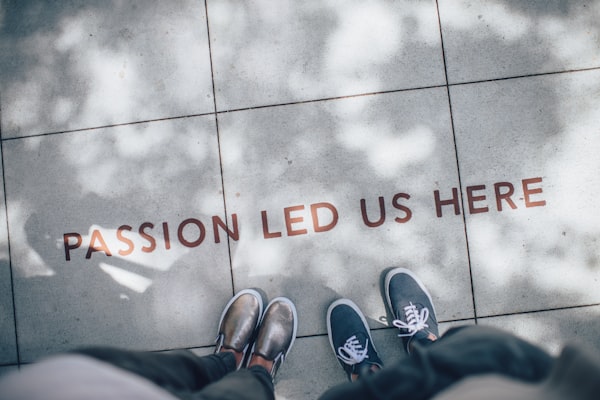
1. 控制器方法的參數未被提供:如果控制器方法聲明瞭一個參數,但是對應的請求沒有包含該參數或者參數名不匹配,就會引發此異常。
2. 前端代碼錯誤:可能是前端的JavaScript代碼或其他邏輯問題導致未能正確地傳遞參數到服務端。
以下是一些有效解決此類異常的方法和建議:
檢查控制器方法和URL映射
首先,確保你的控制器方法中的所有必需參數都已經被明確定義,並且這些參數的名字與你在URL路徑或查詢字符串中使用的名字相匹配。例如,如果你的控制器方法是這樣的:
@GetMapping("/example/{id}")
public String handleExample(@PathVariable Long id, Model model) {
// 處理業務邏輯
}
那麼你的URL應該這樣調用:
GET /example/5 HTTP/1.1
Host: example.com
Accept: application/json
這裏的`5`就是`@PathVariable`註解中指定的`Long id`所需的值。如果你使用的是QueryParam而不是PathVariable,那麼你應該將參數放在查詢字符串中:
GET /example?id=5 HTTP/1.1
Host: example.com
Accept: application/json
驗證前端代碼
其次,要確保前端代碼(如HTML表單、AJAX調用等)已經正確地將所需參數傳送到後端。對於表單提交來說,確保所有的輸入字段都被正確地包含在表單中了;對於AJAX調用來說,確保JSON對象的所有屬性都是有效的。
使用默認值或Optional參數
如果你可以接受某些參數缺失的情況,你可以爲它們提供一個合理的默認值。另外,Spring現在支持使用`Optional`作爲參數類型來指示控制器方法參數的可選性。在這種情況下,如果參數不存在,`Optional`將會返回空而不觸發異常。
自定義異常處理程序
爲了優雅地處理`MissingServletRequestParameterException`和其他類似的異常,你可以創建一個全局的異常處理器類來實現`HandlerExceptionResolver`接口。這允許你對特定類型的異常進行統一的響應處理,比如返回友好的錯誤信息給用戶。
下面是一個簡化的示例實現:
import org.springframework.core.annotation.Order;
import org.springframework.stereotype.Component;
import org.springframework.web.HttpMediaTypeNotSupportedException;
import org.springframework.web.bind.MissingServletRequestParameterException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.servlet.NoHandlerFoundException;
import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler;
import javax.servlet.http.HttpServletRequest;
import java.util.logging.Level;
/**
* 這個類別是用來處理控制器(Controller)可能拋出的例外狀況。
*/
@ControllerAdvice
@Order(-1) // 確保此錯誤處理器優先於其他錯誤處理器執行
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
@Override
protected ResponseEntity<Object> handleMethodArgumentNotValid(MethodArgumentNotValidException ex, HttpHeaders headers, HttpStatus status, WebRequest request) {
return super.handleMethodArgumentNotValid(ex, headers, status, request);
}
@ExceptionHandler({MissingServletRequestParameterException.class})
public ResponseEntity<Object> handleMissingServletRequestParameterException(MissingServletRequestParameterException exception, HttpServletRequest request) {
String message = "請求中缺少必要的參數 '" + exception.getParameterName() + "'。";
return new ResponseEntity<>(new ErrorInfo(message, Level.SEVERE), new HttpHeaders(), HttpStatus.BAD_REQUEST);
}
@ExceptionHandler({HttpMediaTypeNotSupportedException.class})
public ResponseEntity<Object> handleHttpMediaTypeNotSupportedException(HttpMediaTypeNotSupportedException exception, HttpServletRequest request) {
String message = "不支援的媒體類型 '" + exception.getContentType() + "'。";
return new ResponseEntity<>(new ErrorInfo(message, Level.INFO), new HttpHeaders(), HttpStatus.UNSUPPORTED_MEDIA_TYPE);
}
@ExceptionHandler({NoHandlerFoundException.class})
public ResponseEntity<Object> handleNoHandlerFoundException(NoHandlerFoundException exception, HttpServletRequest request) {
String message = "找不到對應的路徑 '" + request.getRequestURI() + "' 的控制項。";
return new ResponseEntity<>(new ErrorInfo(message, Level.WARNING), new HttpHeaders(), HttpStatus.NOT_FOUND);
}
private static final class ErrorInfo {
private final String message;
private final Level level;
ErrorInfo(String message, Level level) {
this.message = message;
this.level = level;
}
public String getMessage() {
return this.message;
}
public Level getLevel() {
return this.level;
}
}
}
通過以上措施,您可以有效地減少`MissingServletRequestParameterException`的出現頻率,併爲您的應用程序的用戶提供更好的體驗。