在Web應用程序開發中,確保用戶輸入數據的正確性和有效性是非常重要的一個環節。C#是一種廣泛使用的服務器端編程語言,而JavaScript則是在客戶端運行的腳本語言,兩者結合使用可以在ASP.NET中實現強大的數據輸入驗證功能。以下是如何通過C#和JavaScript對Web控件進行數據輸入驗證的中文繁體教程:
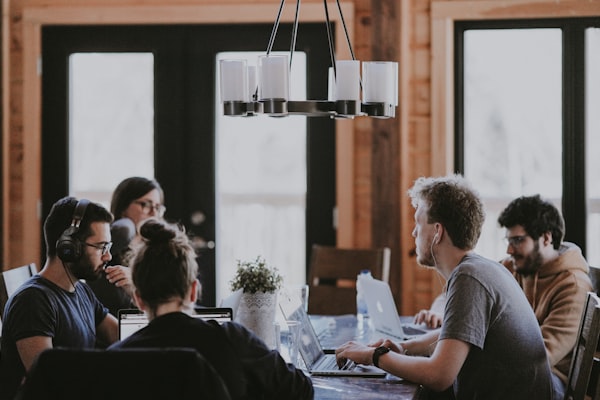
瞭解C#和JavaScript的基本概念
- C#(發音爲“see sharp”)是由微軟開發的面向對象編程語言,常用於Windows Forms應用、ASP.NET web應用和服務器的後端開發。
- JavaScript是一種解釋型腳本語言,可以動態地操作HTML元素、處理事件以及與服務器通信。它是現代網頁不可或缺的一部分。
設置數據輸入驗證的基礎架構
1. 安裝NuGet包 – 在開始之前,請確保已經安裝了最新的[Microsoft Visual Studio](https://visualstudio.microsoft.com/zh-tw/)版本。如果你的項目需要額外的庫或框架來支持數據輸入驗證,你可能需要在Visual Studio中的Package Manager Console中執行以下命令以安裝所需的NuGet包:
Install-Package Microsoft.AspNet.Validation
2. 創建新的ASP.NET項目 – 啓動Visual Studio,選擇”新建項目”並選擇”ASP.NET Web應用程序”類型。選擇你想用的預建模板(例如MVC),然後點擊確定。
3. 配置頁面模型 – 在解決方案資源管理器中找到`Controllers`文件夾,打開`HomeController.cs`文件,添加必要的命名空間和屬性以便訪問數據驗證特性。
4. 設計表單界面 – 在解決方案資源管理器中找到`Views`文件夾,打開對應的視圖文件(通常是`Index.cshtml`或者`Create.cshtml`),將所需的數據輸入字段添加到HTML表單中。
使用C#內置的數據驗證特性
C#提供了多種數據驗證特性,如`RequiredAttribute`, `RangeAttribute`, `RegularExpressionAttribute`等,這些都可以用來裝飾類或屬性的方法參數以強制執行驗證規則。以下是一些示例:
public class PersonModel
{
// RequiredAttribute表示這個字段必須要有值
[Required]
public string Name { get; set; }
// RangeAttribute指定字段的值必須在指定的範圍內
[Range(0, 99)]
public int Age { get; set; }
}
JavaScript客戶端驗證
除了服務器端驗證外,還可以在客戶端使用JavaScript來實現實時數據輸入驗證,這樣可以提高用戶體驗並減少不必要的網絡請求。下面是如何在你的ASP.NET項目中集成客戶端驗證的方法:
1. 添加JQuery引用 – 將jQuery的CDN鏈接添加到你的頁面的“部分:
<script src="https://ajax.aspnetcdn.com/ajax/jquery/jquery-3.5.1.min.js"></script>
2. 啓用客戶端驗證 – 在你的控制器中返回View時,確保啓用了客戶端驗證:
return View().EnableClientValidation(); // 對於ASP.NET MVC
3. 編寫自定義驗證腳本 – 在你的視圖中,你可以使用`data-val`和`data-val-xxx`屬性來指示某個字段是否需要驗證及其相應的錯誤信息。同時,你也可以編寫自己的JavaScript函數來進行更復雜的驗證邏輯。
$(document).ready(function () {
$('#btnSubmit').click(function (e) {
var isValid = true;
$(':input[required=true]').each(function () {
if ($(this).val() === '') {
isValid = false;
}
});
if (!isValid) {
e.preventDefault();
alert('請填寫所有必填項!');
}
});
});
綜合案例演示
爲了更好地理解如何在實際場景中使用C#和JavaScript進行數據輸入驗證,我們來看一個完整的例子。假設有一個註冊表單,我們需要驗證用戶的姓名、電子郵件地址、密碼和確認密碼字段。
首先,我們在C#中定義一個UserModel類,併爲每個字段添加適當的驗證特性:
public class UserModel
{
[Required]
[EmailAddress]
public string Email { get; set; }
[StringLength(16, MinimumLength = 8, ErrorMessage = "Password must be between 8 and 16 characters long.")]
[DataType(DataType.Password)]
public string Password { get; set; }
[Compare("Password", ErrorMessage = "Passwords do not match.")]
[DataType(DataType.Password)]
public string ConfirmPassword { get; set; }
[DisplayName("Full name")]
[Required]
public string FullName { get; set; }
}
接下來,我們將上述代碼中的驗證特性映射到我們的HTML表單字段上:
<div class="form-group">
<label for="email">E-mail address</label>
<input asp-for="Email" type="email" class="form-control" id="email" placeholder="Enter email" required data-val="true" data-val-required="The E-mail Address field is required." />
<span asp-validation-for="Email" class="text-danger"></span>
</div>
<!-- More input fields here with appropriate attributes -->
最後,在我們的JavaScript中,我們可以使用jQuery來監聽提交按鈕的事件,並在客戶端進行驗證:
$(document).ready(function () {
$('#registerForm').submit(function (event) {
var $form = $(this);
$.validator.setDefaults({ errorClass: 'invalid' });
$form.validate({
rules: {
Email: {
required: true,
email: true
},
Password: {
required: true,
minlength: 8,
maxlength: 16
},
ConfirmPassword: {
required: true,
equalTo: '#password'
},
FullName: {
required: true
}
},
messages: {
Email: {
required: 'Please enter your e-mail address.',
email: 'Your e-mail address does not look right.'
},
Password: {
required: '<PASSWORD>',
minlength: 'Password must be at least 8 characters long.',
maxlength: 'Password cannot exceed 16 characters.'
},
ConfirmPassword: {
required: 'Please confirm your password.',
equalTo: 'Passwords do not match.'
},
FullName: {
required: 'Please provide your full name.'
}
}
});
if ($form.valid()) {
event.preventDefault(); // Prevent form submission if validation fails
console.log('Form successfully validated! You can submit it now or handle the result in your own way.');
} else {
console.error('Form validation failed. Check your inputs again.');
}
});
});
通過這種方式,你可以有效地結合C#和JavaScript來確保你的Web應用程序的用戶輸入是安全且有效的。記住,即使有了強有力的數據輸入驗證機制,始終保持警惕並定期更新你的系統以抵禦最新的威脅也是非常重要的。