在Spring框架中,當一個異步請求超過設定的時間限制時,系統會拋出`org.springframework.web.context.request.async.AsyncRequestTimeoutException`這個例外狀況。這通常發生於使用Spring MVC的@Controller或@RestController註解定義的方法被配置為異步執行且未及時完成的情況下。以下是一些解決此問題的可能策略:
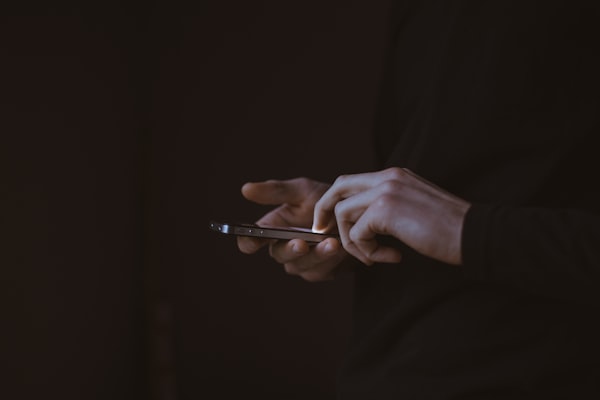
1. 調整異步請求超時設置 – 首先,確認您是否真的需要如此長的等待時間來處理您的請求。如果可以的話,減少超時值可能是一個好的開始。這樣做可以避免無謂的延遲和不必要的資源浪費。例如,可以在Spring應用程式的配置類中使用`WebMvcConfigurerAdapter`接口來實現這一點:
@Configuration
public class WebConfig implements WebMvcConfigurer {
// ... other configuration settings here ...
@Override
public void configureAsyncSupport(final AsyncSupportConfigurer configurer) {
configurer.setDefaultTimeout(Duration.ofSeconds(60)); // 將預設的異步請求超時設定為60秒
}
}
2. 監控異常並進行相應處理 – 在某些情況下,適當地捕捉和處理這個異常可能是最合適的做法。這樣可以使您的程式碼更加健壯,並且能夠提供更友好的錯誤訊息給用戶端:
@Controller
public class MyController {
// ... controller methods go here ...
@Async
public Future<String> longRunningOperation() throws InterruptedException, ExecutionException {
Thread.sleep(5 * 60_000); // 模擬一個耗時5分鐘的操作
return new AsyncResult<>("Long running operation completed successfully!");
}
@GetMapping(value = "/long-running-operation", produces = MediaType.TEXT_PLAIN_VALUE)
public String handleLongRunningOperation(@NonNull final Model model) throws Exception {
try {
Future<String> result = longRunningOperation();
model.addAttribute("message", "Your request is being processed asynchronously. Please wait...");
return "redirect:/waiting";
} catch (InterruptedException | ExecutionException e) {
if (e instanceof ExecutionException && e.getCause() instanceof AsyncRequestTimeoutException) {
throw new RuntimeException("The async task timed out.", e.getCause());
} else if (e instanceof AsyncRequestTimeoutException) {
throw new RuntimeException("The async task timed out.", e);
} else {
throw new RuntimeException("Unexpected error occurred in the async task.", e);
}
}
}
}
3. 重試機制 – 如果可能的話,您可以考慮設計一種重試機制來重新嘗試那些由於超時而被終止的任務。這樣可以防止因單次失敗而丟棄有價值的工作。然而,這種方法應該小心謹慎地使用,因為它有可能導致無窮循環或者惡化已經存在的性能問題:
@Component
public class RetryManager {
private static final int MAX_RETRY_COUNT = 3;
public <T> T retryIfNecessary(Callable<T> callable) {
int retryCount = 0;
while (retryCount <= MAX_RETRY_COUNT) {
try {
return callable.call();
} catch (Exception ex) {
if (retryCount >= MAX_RETRY_COUNT || ex instanceof AsyncRequestTimeoutException) {
break;
}
retryCount++;
}
}
throw new RuntimeException("Maximum number of retries reached or timeout exception encountered.");
}
}
4. 優化和縮短執行時間 – 分析為什麼您的操作需要那麼多時間來完成,並且看看是否有辦法提高其效率。這可能涉及到對數據庫查詢、外部API調用或其他潛在瓶頸的分析和改進。
總之,解決`org.springframework.web.context.request.async.AsyncRequestTimeoutException`異常取決於具體的需求和環境。選擇正確的策略需要對您的應用程式的架構、工作負載和需求有一個深入的理解。