在本文中,我們將探討如何使用IntelliJ IDEA來實現一個前端頁面的登錄、註冊、新增、刪除、修改以及查詢的操作。IntelliJ IDEA是一款功能強大的集成開發環境(Integrated Development Environment, IDE),專爲Java和其他編程語言而設計。它提供了豐富的特性來幫助開發者更高效地編寫代碼和管理項目。以下將逐步介紹如何在IntelliJ IDEA中完成這些任務。
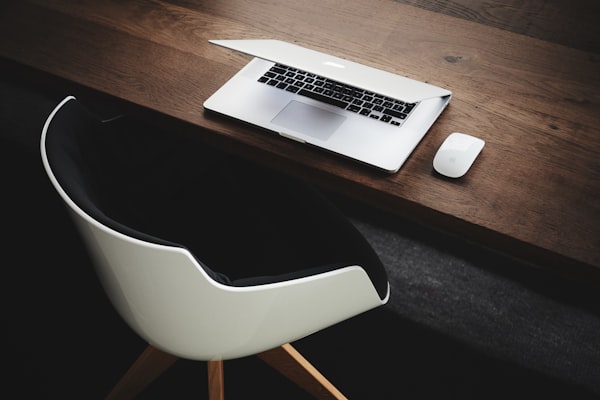
創建新項目
首先,我們需要在IntelliJ IDEA中創建一個新的Web應用程序項目。打開軟件後,選擇“File” > “New Project…”,然後選擇“Web”下的“Web Application”類型。設置項目的名稱和位置,並確保選擇了合適的Java版本和Servlet API的版本。點擊“Next”繼續設置,保持默認的Maven和Tomcat配置即可。最後,點擊“Finish”完成項目的創建。
添加前端框架
爲了快速構建前端界面,我們可以使用流行的JavaScript框架如React或Vue.js。在IntelliJ IDEA中,可以通過安裝插件或者手動導入腳手架的方式來引入這些框架。例如,對於React,你可以通過Settings > Plugins搜索”NodeJS”並安裝該插件,然後重啓IDE以激活插件。接着,運行`npx create-react-app frontend`命令來初始化一個全新的React應用。
編寫登錄和註冊邏輯
接下來,我們開始編寫登錄和註冊的邏輯。這通常涉及到用戶認證和服務端處理兩個部分。在服務端,我們需要驗證用戶的輸入是否合法,比如檢查用戶名是否存在,密碼是否符合複雜度要求等等。如果一切正常,則生成會話令牌(Session Token)或者其他形式的身份標識。在客戶端,我們需要處理錯誤信息並提供友好的提示給用戶。
// Login Form Submission Handler
function handleLoginSubmit(e) {
e.preventDefault(); // Prevent page refresh on form submit
const username = document.getElementById('username').value;
const password = document.getElementById('password').value;
if (!validateUsername(username) || !validatePassword(password)) {
return showErrorMessage('Please enter a valid username and password');
}
fetch('/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
username,
password
})
})
.then((response) => response.json())
.then((data) => {
if (data.success) {
document.cookie = `sessionToken=${data.token}; Max-Age=3600`; // Set session cookie for one hour
window.location.href = '/dashboard'; // Redirect to secured area
} else {
showErrorMessage(data.message); // Show error message from server
}
})
.catch((error) => console.error('Error:', error));
}
// Register Form Submission Handler
function handleRegisterSubmit(e) {
e.preventDefault();
const firstName = document.getElementById('first_name').value;
const lastName = document.getElementById('last_name').value;
const email = document.getElementById('email').value;
const password = document.getElementById('password').value;
if (!validateEmail(email) || !validatePassword(password)) {
return showErrorMessage('Please enter a valid email address and password');
}
fetch('/register', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
firstName,
lastName,
email,
password
})
})
.then((response) => response.json())
.then((data) => {
if (data.success) {
clearFormErrors()
showSuccessMessage('Registration successful. Please login with your new account.')
} else {
showErrorMessage(data.message)
}
})
.catch((error) => console.error('Error:', error));
}
數據持久化和CRUD操作
數據的持久化可以藉助關係數據庫管理系統(RDBMS)如MySQL或者NoSQL數據庫如MongoDB來實現。在IntelliJ IDEA中,我們可以使用其內置的數據庫支持來管理我們的數據庫連接、執行查詢語句以及可視化查看結果。
對於CRUD(Create Read Update Delete)操作,我們可以利用RESTful APIs或者GraphQL接口來與數據庫進行交互。在服務端,我們需要編寫相應的控制器和方法來處理這些請求,並在前端調用這些API來更新UI。
@RestController
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping("/users")
public ResponseEntity<Object> createUser(@RequestBody CreateUserDTO dto) {
User user = userService.createUser(dto);
URI location = ServletUriComponentsBuilder.fromCurrentContextPath().path("/users/{id}").buildAndExpand(user.getId()).toUri();
return ResponseEntity.created(location).build();
}
@GetMapping("/users/{userId}")
public Optional<UserResponseDTO> getUserById(@PathVariable Long userId) {
return userService.getUserById(userId).map(UserMapper::convertToDto);
}
@PutMapping("/users/{userId}")
public void updateUser(@PathVariable Long userId, @RequestBody UpdateUserDTO dto) {
userService.updateUser(userId, mapDTOToEntity(dto));
}
@DeleteMapping("/users/{userId}")
public void deleteUser(@PathVariable Long userId) {
userService.deleteUser(userId);
}
// Utility methods omitted for brevity
}
測試和部署
在開發過程中,不斷地進行單元測試和集成測試是非常重要的。IntelliJ IDEA內置了優秀的測試支持,可以幫助我們輕鬆地編寫和運行測試用例。
當我們的應用程序準備就緒時,可以使用IntelliJ IDEA的工具來打包和部署到生產環境中。這可以是直接發佈到雲平臺,或者通過Docker容器化的方式來簡化部署過程。
IntelliJ IDEA爲我們提供了一個強大且統一的開發環境,幫助我們高效地完成從項目創建到最終發佈的整個流程。無論是前端開發還是後端服務的搭建,都可以在這個平臺上得到很好的支持和優化。