在本文中,我將指導您如何使用HTML、CSS和JavaScript創建一個簡單的促銷頁面,以配合即將到來的618購物節。這個項目旨在展示如何結合這些技術來構建用戶界面,以及如何在實際應用中運用它們。以下是我們將要實現的促銷頁面的基本功能列表:
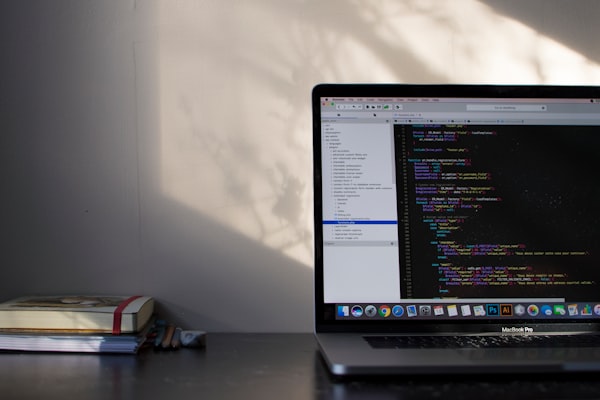
- 首頁Banner廣告
- 產品輪播圖
- 商品分類導航
- 精選商品推薦
- 購物車及結算按鈕
- 動態倒計時計數器
準備階段
首先,我們需要規劃頁面佈局,確定每個部分的位置和大致尺寸。我們可以使用基本的HTML結構來搭建框架,然後通過CSS樣式表定義外觀。對於交互性,我們將在JavaScript中處理。
<!DOCTYPE html>
<html lang="zh-TW">
<head>
<meta charset="UTF-8">
<title>618購物節特別活動</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<!-- Banner Advertisement -->
<h1>618購物節特別活動</h1>
<div id="banner"></div>
<!-- Product Carousel -->
<h2>熱銷產品</h2>
<div id="carousel"></div>
<!-- Category Navigation -->
<h3>分類導航</h3>
<ul class="categories"></ul>
<!-- Featured Products -->
<h4>精選商品</h4>
<div class="featured-products"></div>
<!-- Cart & Checkout Button -->
<h5>我的購物車</h5>
<div class="cart">
<button type="submit">結帳</button>
</div>
<!-- Countdown Timer -->
<p id="countdown">距離優惠截止還剩</p>
<!-- Include JavaScript Files -->
<script src="jquery.min.js" defer></script>
<script src="scripts.js" defer></script>
</body>
</html>
樣式化階段
接下來,我們將添加一些基本的CSS樣式,使頁面看起來更加美觀。這裏只是一個起點,可以根據需要進一步調整顏色、字體和其他細節。
/* Basic Styles */
body {
margin: 0;
padding: 0;
}
h1, h2, h3, h4, h5 {
font-family: Arial, sans-serif;
}
a {
color: inherit;
text-decoration: none;
}
#banner {
width: 100%;
height: auto;
background-image: url('images/banner-ad.jpg');
background-size: cover;
display: flex;
align-items: center;
justify-content: center;
}
#carousel {
width: 100%;
max-width: 960px;
margin: 2em auto;
}
.categories {
list-style: none;
padding: 0;
}
.categories li + li {
margin-top: 1em;
}
.featured-products {
margin: 2em 0;
}
.cart button {
border: none;
padding: 0.75em 1.5em;
background-color: #ffc400;
color: white;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
.cart button:hover {
opacity: 0.9;
}
#countdown {
font-weight: bold;
}
行爲與互動
現在,讓我們用JavaScript給頁面增加一點活力。我們將會用到jQuery庫,因爲它可以簡化DOM操作和動畫效果的編寫。下面是如何實現一些基本功能的示例腳本:
// Load jQuery (You may need to include it from a CDN or download the latest version)
$(function() { // DOM ready event handler
// Display banner content with jQuery's HTML method
$('#banner').html('<img src="images/banner-ad.jpg" alt="618 Shopping Festival Ad" />');
// Set up product carousel using any available plugin or create your own
// Create category navigation items dynamically
var categories = ["Electronics", "Fashion", "Books"];
for (let i = 0; i < categories.length; i++) {
$('.categories').append(`<li><a href="#">${categories[i]}</a></li>`);
}
// Generate featured products list and add click events for each item
// Initialize countdown timer with moment.js and update every second
var endDate = new Date("2023-06-18T00:00:00Z"); // Replace this with actual date
setInterval(function () {
$('#countdown').html(moment.utc(endDate - new Date()).format('dd[天] HH[小時] mm[分鐘] ss[秒]'));
}, 1000);
});
請注意,以上代碼僅爲示例目的,具體實現可能會涉及到更多複雜的邏輯和設計決策。在實際項目中,你可能還需要考慮性能優化、響應式設計和更豐富的交互體驗。