在現代Web開發中,前端技術日新月異,開發者需要不斷地學習和更新自己的知識以適應新的需求和技術發展。其中,前端數據處理和報表生成功能變得越來越重要,特別是在商業應用和企業系統中。本文將探討如何使用Vue.js 3框架結合Excel文件格式來實現一個靈活的前端數據導出功能。
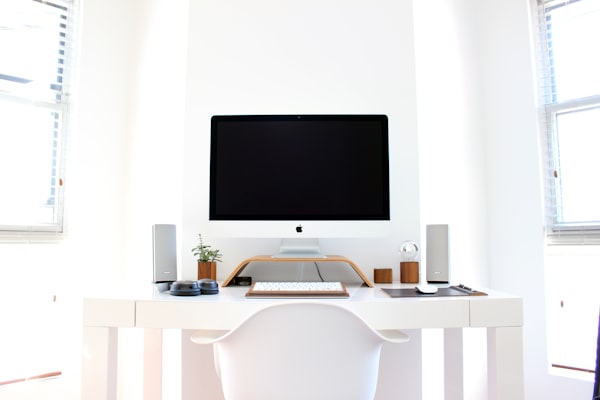
Vue.js簡介
Vue.js是一款流行的漸進式JavaScript框架,它提供了簡潔、高效且易於學習的API來構建用戶界面。Vue.js的響應性系統使得狀態變化時自動更新DOM成爲可能,這極大地簡化了開發工作流。
Excel文件格式概述
Microsoft Excel是一種廣泛使用的電子表格軟件,其文件格式(XLSX)是基於XML的ZIP壓縮文件。這種格式的優點之一是可以通過編程方式輕鬆地創建、讀取和修改Excel文檔。
實現Excel導出的步驟
1. 安裝依賴包
首先,我們需要通過npm安裝以下兩個關鍵庫:`xlsx` 和 `xlsx-style`。這兩個庫可以幫助我們更方便地操作Excel文件:
npm install xlsx xlsx-style --save
2. 在Vue項目中集成`xlsx`和`xlsx-style`
接下來,我們將這些庫導入到我們的Vue項目中。假設我們已經有一個基於Vue.js 3的項目,我們可以這樣引入這些模塊:
// main.js or similar entry point to your application
import XLSX from 'xlsx'; // Import the core Excel file manipulation library
import { Stylesheet, Style } from 'xlsx-style'; // Import additional styling capabilities
const workbook = new XLSX.Workbook(); // Create a new Workbook instance
let styles: any; // Declare an empty object for our custom styles
if (process.env.NODE_ENV === 'production') {
styles = new Stylesheet({ debug: false }); // Initialize the stylesheet with debugging disabled in production
} else {
styles = new Stylesheet({ debug: true }); // Enable debugging during development
}
3. 定義數據模型和模板
爲了使數據可導出爲Excel文件,我們需要定義數據的結構以及如何在Excel中呈現它們。這可能涉及到定製單元格的樣式、合併單元格、設置字體大小和顏色等等。以下是一些示例代碼段:
// Define data model and template structure
export interface DataRow {
id: number;
name: string;
age: number;
city: string;
}
interface TemplateOptions {
sheetName?: string; // Name of the sheet where data will be exported
headerRows?: number[]; // Rows that should be considered as headers
dataStartRow?: number; // Row index where actual data starts
columns: { [key: string]: Column }; // Map of column definitions
}
type Column = {
field: keyof DataRow; // Property name on the data row object
title: string; // Title/label for this column
width?: number | null; // Optional width override in pixels
};
4. 實現數據導出邏輯
現在,我們可以編寫函數來實際執行數據導出了:
// Export function implementation using Vue's composition API (using setup() pattern)
setup(props, context) {
function exportDataToExcel(rows: DataRow[]): void {
// Prepare worksheet by adding header rows if defined
for (let i = 0; i < rows.length - 1; i++) {
if (i >= props.template.headerRows!.length && i > props.template.dataStartRow!) break;
const currentRow = rows[i];
const wsRow: XLSX.WSRow = [];
for (const columnName in props.template.columns) {
wsRow.push(currentRow[columnName]);
}
workbook.addWorksheet().addRow(wsRow, { align: 'center' });
}
// Apply custom styles to the worksheet cells
for (const cell of props.template.columns) {
const styleObj: Partial<Style> = {};
if (cell.width) {
styleObj['sz'] = `${cell.width}`;
}
styles.addCellStyle('custom-' + cell.field, styleObj);
}
// Iterate over each row and apply styles based on field names
for (let r = 0; r <= rows.length - 1; ++r) {
const currentRow = rows[r];
for (const columnName in props.template.columns) {
const cellValue = currentRow[columnName];
if (cellValue == undefined || cellValue == null) continue;
const styleKey = 'default';
if (typeof cellValue === 'number' || typeof cellValue === 'boolean') {
styleKey = 'num';
} else if (typeof cellValue === 'string') {
switch (true) {
case /^[-+]?\d+\.?\d*$/.test(cellValue):
styleKey = 'num';
break;
case /^\w+$/..test(cellValue):
styleKey = 'txt';
break;
default:
styleKey = 'str';
}
}
if (r === 0 && props.template.columns[columnName].hasOwnProperty('width')) {
styles.setColumn(props.template.columns[columnName]['width'], 'A');
}
styles.applyCellStyle(r, 'A', styleKey);
}
}
// Save the workbook to a blob and trigger download
const excelBuffer: BlobPart = XLSX.write(workbook, { bookType: 'xlsx', type: 'array' });
const excelBlob = new Blob([excelBuffer], { type: "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet" });
context.$emit('download', URL.createObjectURL(excelBlob));
}
return {
exportDataToExcel, // Expose the export method to the component
};
}
5. 在Vue組件中調用導出方法
最後,我們在Vue組件中提供一個按鈕或觸發器來調用上述的導出方法:
<!-- Vue Component template -->
<template>
<div class="container">
<h1>Export Data</h1>
<p>Click below to export data into an Excel spreadsheet</p>
<button @click="onDownloadExcel">Download Excel</button>
</div>
</template>
<script lang="ts">
export default {
methods: {
async onDownloadExcel(): Promise<void> {
this.exportDataToExcel(/* Your data array goes here */);
},
},
};
</script>
請注意,上述代碼只是一個高級概念性的指南,實際的實現可能會更加複雜,並且會根據具體的需求而有所不同。在實際項目開發中,你可能還需要考慮錯誤處理、性能優化、國際化支持以及其他特定的業務需求。