在 Spring Boot 中,前端可以通過表單將數據以數組的形式發送到後端。後端可以使用不同的方式來處理這些數據,這取決於前端的具體實現以及數據的格式。以下是一些常見的策略和方法:
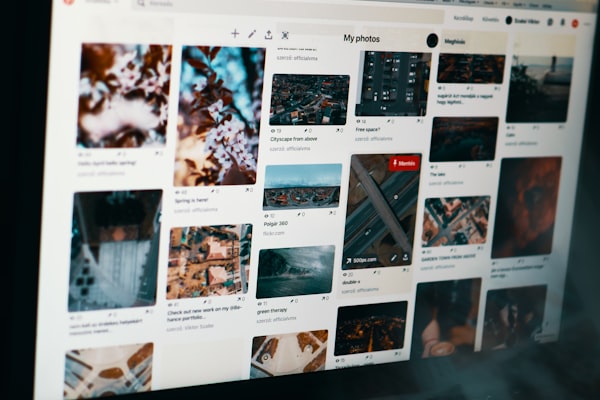
1. 使用`@RequestParam`註解
如果前端通過查詢參數(query parameter)的方式發送數據,並且每個數組項都是單獨的參數,那麼你可以使用 `@RequestParam` 註解來獲取它們。例如,假設前端發送的數據是這樣的:
http://example.com/some-endpoint?item1=value1&item2=value2&item3=value3
後端控制器方法可以這樣定義:
public class MyController {
@GetMapping("/some-endpoint")
public ResponseEntity<String> handleRequest(@RequestParam("item1") String item1, @RequestParam("item2") String item2, @RequestParam("item3") String item3) {
// Process the items here
}
}
2. 使用`@PathVariable`註解
如果你正在處理的是RESTful API中的路徑變量,你可以使用 `@PathVariable` 註解。在這種情況下,每個數組項都是一個路徑的一部分。例如,假設前端發送這樣的請求:
http://example.com/items/item1/item2/item3
後端控制器方法可以這樣定義:
public class MyController {
@GetMapping("/items/{item1}/{item2}/{item3}")
public ResponseEntity<String> handleRequest(@PathVariable("item1") String item1, @PathVariable("item2") String item2, @PathVariable("item3") String item3) {
// Process the items here
}
}
3. 使用`@ModelAttribute`註解
如果你的前端是通過HTML表單發送數據,並且使用的是傳統的多部分/文件上傳模式,你可以使用 `@ModelAttribute` 註解來綁定數據到一個對象上。例如,假設前端發送一個包含數組的JSON字符串:
{ "myArray": ["value1", "value2", "value3"] }
後端控制器方法可以這樣定義:
public class MyController {
@PostMapping("/some-endpoint")
public ResponseEntity<String> handleRequest(@ModelAttribute SomeDTO dto) {
List<String> array = dto.getMyArray(); // Get the list from the DTO object
// Process the array here
}
}
其中`SomeDTO`是一個帶有屬性`myArray`的對象。這個屬性的類型應該與前端發送的數據相匹配。
4. 直接從HTTP請求中獲取數據
有時候你可能需要直接訪問HttpServletRequest對象,以便讀取如`MultiValueMap`或`Part[]`類型的數據。這種情況下,你可以像下面這樣做:
public class MyController {
@PostMapping("/some-endpoint")
public ResponseEntity<String> handleRequest(MultipartHttpServletRequest request) {
List<String> myArray = new ArrayList<>();
for (Iterator<String> itr = request.getFileNames(); itr.hasNext(); ) {
String fieldName = itr.next();
myArray.add(request.getParameter(fieldName));
}
// Process the array here
}
}
5. 使用Spring WebFlux
對於Reactive應用程序,Spring WebFlux提供了類似的方法來處理數組數據。例如:
import org.springframework.web.reactive.function.server.ServerRequest;
import reactor.core.publisher.Mono;
public Mono<Void> handleRequest(ServerRequest serverRequest) {
List<Integer> numbers = serverRequest.queryParam("numbers").mapToInt(Integer::parseInt).collectList().blockOptional().orElse(null);
// Do something with 'numbers' list
return Mono.empty();
}
在實際開發過程中,你應該選擇最符合你應用需求的方法。這可能涉及到性能考慮、API設計規範或者其他技術因素。無論哪種情況,理解如何正確地從前端獲取數據並將它映射到你的業務邏輯是非常重要的。