在Spring框架中,HandlerInterceptor接口提供了一個機會來擴展控制器(Controller)的方法執行過程。通過實現這個接口,你可以定義一個攔截器類,它會在請求被處理之前或之後觸發某些邏輯。以下是如何使用HandlerInterceptor的簡要指南:
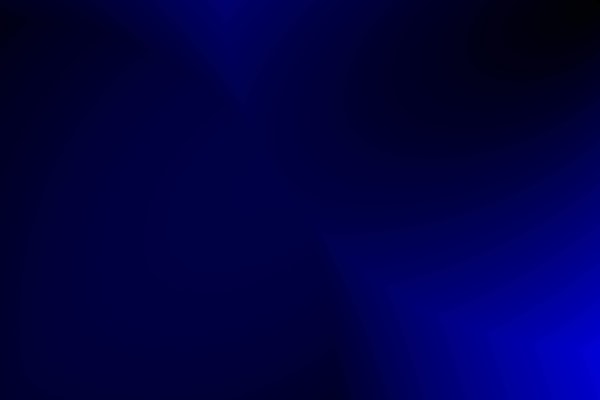
1. 創建攔截器:首先,你需要創建一個類來實現`HandlerInterceptor`接口。該接口有兩個方法需要重寫:`preHandle()`, `postHandle()` 和 `afterCompletion()`。這些方法是可以在請求生命週期中的不同點執行的鉤子函數。
public class MyHandlerInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
// 在控制器方法被調用前執行的操作
System.out.println("Pre-handle called!");
return true; // 如果返回false,則阻止請求繼續執行
}
@Override
public void postHandle(HttpServletRequest request, HttpservletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
// 在控制器方法執行後,視圖渲染前執行的操作
System.out.println("Post-handle called!");
}
@Override
public void afterComplation(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
// 整個請求處理完成後執行的操作
System.out.println("After completion called!");
}
}
2. 配置攔截器:接下來,你需要在你的Spring應用程序配置文件中註冊你的攔截器。這可以通過多種方式完成,但最常見的方式是通過`WebMvcConfigurer`接口的`addInterceptors()`方法。
3. WebMvcConfigurer接口:`WebMvcConfigurer`接口提供了許多用於配置Spring MVC的功能。例如,你可以使用它的`addInterceptors()`方法添加一個或多個`HandlerInterceptor`實例到你的應用程序中。
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
private final MyHandlerInterceptor myInterceptor; // 假設這是一個已經創建好的MyHandlerInterceptor對象
// ...其他配置代碼...
/**
* 將我們的自定義HandlerInterceptor添加爲全局攔截器
*/
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(myInterceptor)
// 指定哪些URL路徑將被攔截
.addPathPatterns("/path_to_be_intercepted/*")
// 設置排除的路徑,即不被攔截的路徑
.excludePathPatterns("/excluded_path/*");
}
}
在上述示例中,我們配置了`myInterceptor`攔截所有以`/path_to_be_intercepted/`開頭的URL路徑,同時排除`/excluded_path/*`路徑。
4. 整合Spring Boot:如果你在使用Spring Boot,你可以將上述配置放入到一個`@Configuration`註解的類中,並將它作爲Bean註冊。這樣就可以讓Spring IoC容器管理你的攔截器的生命週期。
請注意,這裏只是一個簡單的概念性解釋,實際的開發環境中可能還需要考慮更多細節,比如異常處理、安全性和性能優化等等。在實際項目中,你應該根據需求定製合適的解決方案。