在現代Web應用中,前後端的分離是一種常見的架構模式,其中涉及到的關鍵技術點之一是如何在前端處理後端返回的二進制數據,例如圖像文件。本文將探討如何在Vue.js前端顯示從後端獲取的文件流,以及相關的最佳實踐和安全注意事項。
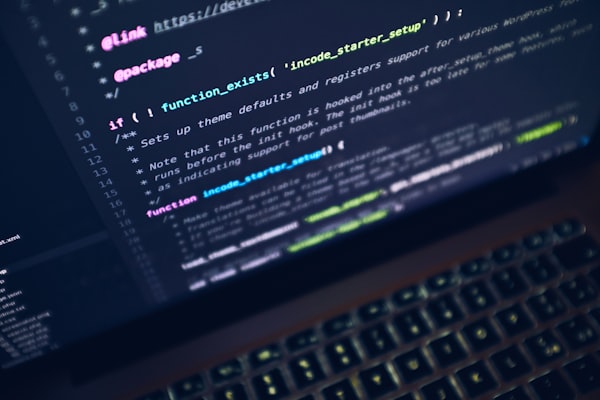
1. 理解HTTP協議中的Content-Type
爲了正確地處理不同類型的資源,如文本、HTML頁面或圖像,HTTP協議使用了一個稱爲`Content-Type`的頭字段來指定資源的MIME類型。對於圖像文件來說,常見的MIME類型有`image/jpeg`, `image/png`, `image/gif`等等。
2. 在後端生成文件流
在後端服務器上,你可以通過多種方式生成文件流,這取決於你的應用程序所使用的編程語言和技術棧。以下是如何用不同的後端語言實現這一功能的示例代碼片段:
Python (Flask)
from flask import Flask, send_file
app = Flask(__name__)
@app.route('/download', methods=['GET'])
def download():
# 假設你已經有了要發送給前端的文件對象
with open('example.jpg', 'rb') as file:
return send_file(file, attachment_filename='example.jpg', as_attachment=True)
Node.js (Express)
const express = require('express');
const fs = require('fs');
const app = express();
app.get('/download', function(req, res){
// 打開文件
var readStream = fs.createReadStream('example.jpg');
readStream.on('open', function() {
res.set({
'Content-Disposition': 'attachment; filename*="example.jpg"'
});
readStream.pipe(res);
});
});
Java (Spring Boot)
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class FileDownloadController {
@GetMapping(value = "/downloadFile")
public ResponseEntity<Resource> downloadFile() {
Resource resource = new ClassPathResource("images/example.jpg"); // 假設這是一個有效的路徑到你的資源
return ResponseEntity.ok()
.contentLength(resource.contentLengthLong())
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
}
3. Vue.js前端處理文件流
在前端,你可以使用JavaScript原生的`fetch API`或者Axios庫來請求這些資源。一旦接收到響應,你需要檢查`Content-Type`頭字段以確定它是普通的JSON響應還是文件流。如果是文件流,則需要將其下載到本地存儲或直接在瀏覽器中進行渲染。
Fetch API
async function downloadImage() {
try {
let response = await fetch('/download'); // 將'/download'替換爲實際的URL
if (response.headers.get('Content-Type').startsWith('image')) {
// 如果頭部是圖像類型,我們可以創建一個新的Image對象
let blob = await response.blob();
let urlCreator = window.URL || window.webkitURL; // URL接口在不同瀏覽器的兼容性處理
let imageUrl = urlCreator.createObjectURL(blob);
document.getElementById('myImage').src = imageUrl; // #myImage是一個已經存在於DOM中的img元素
} else if (response.ok && response.status === 200) {
// 如果不是圖像流,那麼它可能是其他類型的JSON響應
console.log('Success:', response.json());
} else {
throw Error(response.statusText);
}
} catch (error) {
console.error('Error:', error);
}
}
Axios
axios.interceptors.response.use((response) => {
if (response.headers['content-type'].indexOf('image') > -1) {
// 這裏我們假設我們已經有一個名爲'MyComponent'的組件,並且它包含一個<img>元素
this.$refs.MyComponent.showImage(response.data);
}
return response;
}, (error) => {
// handle error
})
methods: {
showImage(data) {
// 假設data是一個Blob對象
const reader = new FileReader();
reader.addEventListener('loadend', () => {
// 現在,我們將base64編碼的字符串賦值給src屬性
this.$el.querySelector('#myImage').src = reader.result;
});
reader.readAsDataURL(data);
}
}
4. 安全考慮
在處理文件流時,請注意以下幾個安全問題:
- 跨站腳本攻擊(XSS):確保在你的前端代碼中對任何用戶提供的數據進行適當的安全處理,避免惡意腳本被執行。
- CSRF防護:如果你的應用涉及到敏感操作,比如上傳或修改文件,確保你有適當的措施來防止跨站請求僞造(CSRF)。
- 訪問控制:確保只有授權的用戶可以訪問特定的文件資源。
5. 性能優化
- 壓縮文件:在使用之前,對圖像和其他媒體文件進行適當的壓縮以減少其大小,這將有助於提高加載速度。
- 緩存策略:設置正確的HTTP緩存策略,以便客戶端可以有效地緩存靜態資源。
- CDN部署:如果可能,使用內容分發網絡(CDN)來加快資源傳輸速度。
6. 總結
在後端返回一個文件流並在前端Vue.js應用中顯示,需要考慮到多個方面的問題,包括HTTP協議規範、後端編程技巧、前端JavaScript處理以及安全性與性能的最佳實踐。通過遵循上述步驟和建議,您可以成功地在您的Web應用程序中集成這個功能。