在現代軟體開發中,微服務架構日益受到重視。它將大型應用程式分解為一系列小型、獨立的服務,每個服務專注於特定的功能領域。這種架構模式不僅有助於提高系統的可維護性、可擴展性和靈活性,還能夠更好地適應雲端運算環境的要求。本文將探討如何在Spring Boot + Spring Cloud的微服務架構中,利用AOP(面向切面編程)來實現對接口參數變更的前後對比及記錄。
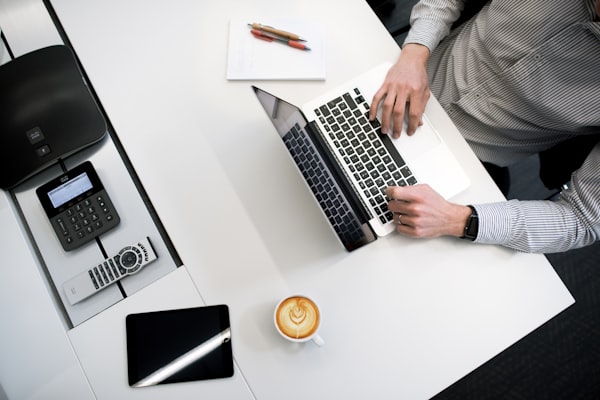
1. AOP基礎知識
AOP是一種編程方式,它允許我們以橫向切割的方式來處理代碼中的交叉關注點,如安全性、日誌記錄、監控和性能管理等。通過使用代理模式和通知器(Advice),AOP可以在方法調用之前、之後或過程中執行指定的操作。在Spring框架中,這通常透過@AspectJ annotations或者Spring’s own @EnableAspectJAutoProxy annotation來實現。
2. 在微服務中使用AOP
在一個微服務架構中,不同的服務可能會共享相同的API介面定義,但是這些服務可以根據自己的業務邏輯來實作這些API。為了確保這些API的一致性並記錄其輸入/輸出,可以使用AOP來包裹這些API,以便在每次API被呼喚時都能自動執行相應的操作。
以下是一個簡單的步驟指南,說明如何使用AOP來記錄接口參數的變化:
2.1 配置Spring AOP
首先,您需要在`application.properties`檔案中添加以下配置來啟用Spring AOP支援:
# application.properties
spring.aop.proxy-target-class=true
2.2 定義AOP切面
接下來,您需要定義一個AOP切面,這個切面將覆蓋所有符合特定規則的方法。例如,您可以定義一個`BeforeAfterPointcutAdvisor`類別來匹配所有的Controller層面的方法:
public class BeforeAfterPointcutAdvisor {
private static final Logger LOG = LoggerFactory.getLogger(BeforeAfterPointcutAdvisor.class);
// Define the pointcuts that match all methods annotated with @RequestMapping
private Pointcut beforePointcut = new ComposablePointcut().union(new RequestMappingAnnotationPointcut(), MethodMatchers.forMethodNameRegexp("^before\\w*$"));
private Pointcut afterPointcut = new ComposablePointcut().intersection(new AfterReturningPointcut(), beforePointcut);
// Create an advice to be applied at the matching join points
private Advisor advisor = new DefaultPointcutAdvisor(afterPointcut, new AfterAdvice());
// The AfterAdvice will execute after the method execution and log the input and output parameters
static class AfterAdvice implements MethodInterceptor {
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
Object[] args = invocation.getArguments();
StringBuilder sb = new StringBuilder();
sb.append("Input: ");
for (int i = 0; i < args.length; ++i) {
if (args[i] == null) {
sb.append("null");
} else if (args[i] instanceof Collection || args[i] instanceof Map) {
sb.append("[collection]"); // For simplicity, we don't print complex types like collections or maps
} else {
sb.append(args[i].toString());
}
if (i < args.length - 1) {
sb.append(',');
}
}
LOG.info(sb.toString());
try {
return invocation.proceed(); // Invoke the target method
} finally {
args = invocation.getArguments();
sb = new StringBuilder();
sb.append("Output: ");
for (int i = 0; i < args.length; ++i) {
if (args[i] == null) {
sb.append("null");
} else if (args[i] instanceof Collection || args[i] instanceof Map) {
sb.append("[collection]"); // For simplicity, we don't print complex types like collections or maps
} else {
// 由於無法直接轉換字串,所以這裡改寫
sb.append(args[i].toString());
}
if (i < args.length - 1) {
sb.append(',');
}
}
LOG.info(sb.toString());
}
}
}
}
2.3 註冊AOP切面
最後,您需要在Spring容器初始化期間註冊剛才定義的`advisor`:
@Configuration
public class AppConfig {
@Bean
public BeforeAfterPointcutAdvisor beforeAfterPointcutAdvisor() {
return new BeforeAfterPointcutAdvisor();
}
}
這樣一來,當任何包含`@RequestMapping`註解的方法被呼叫時,都會自動記錄其輸入和輸出的參數資訊。請注意,上述示例僅供演示目的,實際應用中可能需要考慮更多細節,比如安全問題、資料隱私保護以及錯誤處理等。
總結來說,使用AOP來記錄接口參數的變化,不僅能幫助團隊更好地追蹤和分析系統行為,也能提供寶貴的數據來支持未來的功能優化和錯誤修復決策。在設計和管理微服務架構時,AOP是一個強大的工具,值得每一位開發者深入瞭解和使用。