在Vue.js框架中,要實現播放RTSP(Real-Time Streaming Protocol)視頻流的功能,通常需要藉助第三方插件或庫來處理。以下是一些步驟和建議,幫助您在前端使用Vue.js播放RTSP視頻流:
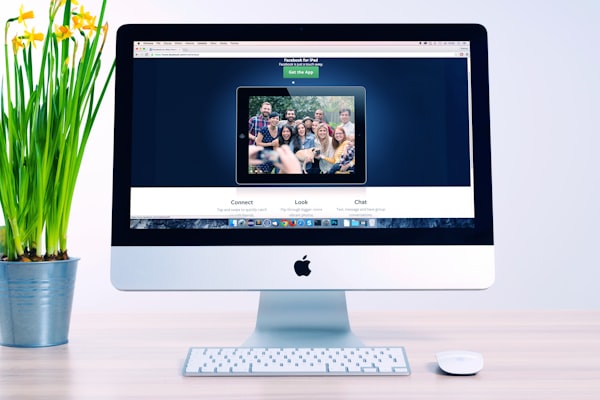
1. 選擇合適的播放器:
市場上有很多開源的媒體播放器可以支持RTSP視頻流的播放,例如Video.js、hls.js、Shaka Player等等。這些播放器可能需要額外的配置或者編碼工作才能正確地播放RTSP流。
2. 安裝必要的依賴包:
在使用任何第三方庫之前,首先需要在您的項目中安裝相應的npm模塊。以下是如何使用`video.js`作爲示例:
# Using npm
npm install video.js @videojs/http-streaming --save
3. 設置HTML結構:
在您的Vue組件的模板部分添加一個 Video.js 容器和一個 “ 元素,用於指定 RTSP URL:
<!-- Your Vue Component Template -->
<template>
<div class="media-player">
<video id="example-video" class="video-js vjs-default-skin" controls preload="auto" width="640" height="264" poster="your_poster.jpg" data-setup='{"techOrder": ["vhs"]}'>
<source :src="rtspUrl" type="application/x-rtsp-stream" />
Your browser does not support the HTML5 Video element.
</video>
</div>
</template>
注意:確保`data-setup`屬性中的`techOrder`數組包含`”vhs”`(Video Home System),這是Video.js對HLS的支持。
4. 處理RTSP URL:
在Vue組件的JavaScript代碼中,獲取RTSP URL並在適當的時候更新它。這可能涉及從服務器獲取數據或者直接硬編碼URL到組件中。
5. 加載視頻源:
當您的應用程序準備就緒時,調用Video.js的方法以加載視頻源並開始播放:
// Inside your Vue component's methods
methods: {
playRTSPRecord() {
var player = videojs('example-video'); // Get a reference to the Video.js player
if (player && player.readyState() === player.HAS_METADATA) {
console.log('Player is ready and has metadata!');
player.loadSource(this.rtspUrl); // Load the RTSP stream URL
player.on('loadedmetadata', function () {
console.log('Metadata loaded!');
player.play(); // Play the video when the metadata is available
});
} else {
console.warn('Player not ready or no metadata present');
}
},
}
請記住,上述代碼只是一個簡化的例子,實際應用中可能會有更多複雜的邏輯和錯誤處理。此外,由於安全原因,許多瀏覽器限制了訪問RTSP流的能力,因此可能需要在服務端通過代理或者其他方式轉換爲HTTP格式再進行播放。