在開始學習Spring Web MVC框架之前,首先需要了解一些基礎概念和背景知識。Spring Web MVC是基於Spring Framework的一個模塊,它提供了一種靈活且可擴展的模型-視圖-控制器(MVC)架構來開發Web應用程序。該框架可以幫助開發者構建高效、可維護性和可測試性的Web應用。以下是Spring Web MVC的簡要介紹以及如何快速上手使用該框架:
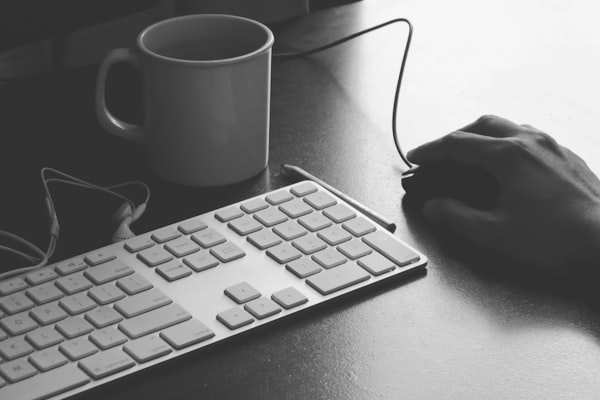
Spring Web MVC簡介
1. 什麼是Spring Web MVC?
Spring Web MVC是一種用於創建Web應用程序的強大框架,它是Spring Framework的一部分。它提供了一個控制反轉容器(IoC)和一個基於AOP的面向切面編程支持。通過Spring Web MVC,開發者可以輕鬆地處理HTTP請求和響應,並將業務邏輯與表示層分離。
2. 爲什麼選擇Spring Web MVC?
Spring Web MVC提供了以下優勢:
- 非侵入性設計:控制器類不需要繼承特定的基類或實現特定接口,它們可以是普通的POJO(Plain Old Java Objects)。
- 易於配置:可以通過簡單的XML文件或者註解來配置控制器和方法映射。
- 強大的異常處理機制:可以定義全局統一的異常處理方式。
- 支持多種數據綁定技術:例如使用@RequestParam, @PathVariable, @ModelAttribute等註解進行參數綁定。
- 國際化支持:通過資源 bundles 和 JSTL 標籤庫來實現國際化的消息傳遞。
- 內置驗證功能:使用JSR-303 Bean Validation標準來進行對象屬性的校驗。
3. Spring Web MVC的主要組件
Spring Web MVC主要由以下幾個主要組件構成:
- DispatcherServlet: 是整個流程的核心,負責接收HTTP請求並將它們分發給相應的處理器處理。
- HandlerMapping: 負責將請求映射到具體的Controller方法。
- Controller (或稱Command/Service): 處理用戶輸入並返回一個ModelAndView對象,其中包含了要展示的數據和使用的模板名。
- ModelAndView: 封裝了將要被渲染的模型數據及用來顯示這些數據的視圖名。
- ViewResolver: 將視圖名解析爲真正的View實例,通常會使用InternalResourceViewResolver將視圖名轉換爲JSP頁面路徑。
Spring Web MVC快速入門
下面我們將按照步驟指導您如何在項目中集成和使用Spring Web MVC:
Step 1: 設置項目環境
確保您的IDE(如IntelliJ IDEA, Eclipse等)已經安裝並且可以正常工作。然後新建一個Maven或Gradle項目,並添加必要的依賴項,如下所示:
<dependencies>
<!-- Spring Core and other spring modules -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.x.y</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.x.y</version>
</dependency>
<!-- Other dependencies like Apache Commons Lang, Jackson for JSON processing etc. can also be added here -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Step 2: 配置Spring上下文
在`src/main/resources`目錄下創建一個名爲`applicationContext.xml`的文件,在其中添加以下內容以配置Spring IoC容器:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- Enable annotation based controllers -->
<mvc:annotation-driven />
<!-- Configure view resolution -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:prefix="/WEB-INF/views/" p:suffix=".jsp"/>
<!-- Define your beans here e.g. DataSource bean, Service classes etc. -->
</beans>
Step 3: 編寫控制器類
接下來,我們需要創建一個控制器類來處理HTTP請求。這個類應該聲明爲`@Controller`,幷包含帶有`@RequestMapping`註解的方法。下面的例子演示了一個簡單的Hello World控制器:
package com.example;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
// Import the necessary annotations from Spring's web module
import static org.springframework.web.bind.annotation.RequestMethod.GET;
@Controller // This tells Spring that this is a Controller class
public class HelloWorldController {
@RequestMapping(value = "/hello", method = GET) // Map HTTP GET requests to /hello URL
public String handleRequest(ModelMap model) { // ModelMap is used to pass data to the view
model.addAttribute("message", "Hello World!"); // Add an attribute to the model
return "helloWorld"; // Return the name of the view to render
}
}
Step 4: 創建視圖
現在我們還需要提供一個JSP頁面來作爲我們的視圖。在`src/main/resources/templates`目錄下創建一個名爲`helloWorld.jsp`的文件,並添加以下內容:
<h1>${message}</h1>
Step 5: 打包和部署WAR文件
最後,我們需要將項目打包成一個WAR文件並在服務器上部署它。這通常涉及到配置Tomcat或其他任何兼容的 servlet 容器。
小結
以上就是Spring Web MVC的基本入門指南。在實際的項目中,你可能還會遇到更多複雜的場景,比如攔截器、表單驗證、國際化、安全認證等等。不過,有了這些基本的認識後,你可以繼續深入研究Spring Web MVC的各個方面,並通過實踐來提高自己的技能水平。