在現代Web開發中,Vue.js 是一種非常受歡迎的JavaScript框架,它提供了強大的功能來建立用戶界面和單頁應用程式(SPAs)。 Vue.js 的最新版本 Vue3 和快速開發環境 Vite 組合在一起可以提供更高效的工作流程和更好的性能。然而,當您嘗試使用 `require` 在 Vue3 + Vite 專案中引入第三方模塊時,可能會遇到 “`require is not defined`” 的錯誤訊息。這篇文章將探討這個問題的原因和解決方法。
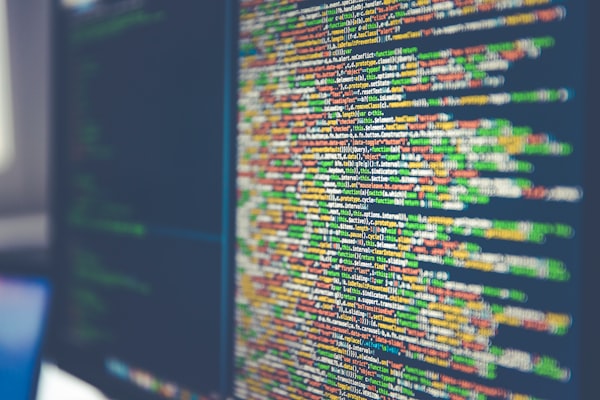
問題分析
當出現 “`require is not defined`” 的錯誤時,通常意味著您的代碼是在瀏覽器環境下運行的,但是使用了 Node.js 風格的模塊系統 (CommonJS) 中的 `require()` 函數。在瀏覽器環境中,並沒有預設定義 `require` 函數,因為瀏覽器的模塊系統遵循的是 ECMAScript Modules (ESM) 標準。而 Vite 是基於 ESBuild 等工具構建的,主要針對瀏覽器環境進行優化,因此它不會自動轉換或封裝 CommonJS 模塊以使其能在瀏覽器中工作。
解決方案
要解決這個問題,您可以採用以下幾種策略:
1. 使用 ESM 兼容的包
首先,檢查您正在使用的第三方庫是否已經有 ESM 版本的供應。許多流行的庫現在都提供了一個 ESM 入口點,這樣就可以直接通過 `import` 語句引入而不需要任何額外的配置。如果存在這樣的版本,請考慮改用該版本。
例如,假設您想要引入一個名為 `my-library` 的庫,並且發現它在 `package.json` 中具有 `main: ‘lib/index.cjs’` 和 `module: ‘esm/index.js’` 屬性。那麼您應該選擇從 `esm/index.js` 導入,因為它是為 ESM 設計的,可以直接在瀏覽器中使用。
// 在 Vue3 + Vite 專案的文件中
import MyLibrary from 'my-library'; // 使用 ESM 入口點
console.log('MyLibrary:', MyLibrary);
2. 使用 Babel 插件或 Polyfill
如果您必須使用不支援 ESM 的舊版庫或者無法輕易替換的庫,可以使用 Babel 插件如 `@babel/plugin-transform-modules-commonjs` 來將 CommonJS 模塊轉譯為可用的 ESM。此外,您還可以在根層級的 `vite.config.ts` 中添加對 `node_modules` 目錄的 polyfill,如下所示:
// vite.config.ts
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
export default defineConfig({
plugins: [react()],
resolve: {
alias: {
'~': `${process.cwd()}/src/`,
},
fallback: {
path: require.resolve('path-browserify'),
fs: false,
util: require.resolve('util-deprecate'),
os: '',
crypto: '',
stream: require.resolve('readable-stream'),
assert: '',
http: '',
https: '',
zlib: '',
url: require.resolve('url/'),
buffer: require.resolve('buffer/'),
vm: require.resolve('vm-browserify'),
querystring: require.resolve('querystring-es3'),
tty: require.resolve('tty-browserify'),
dns: require.resolve('dns.js'),
net: require.resolve('net.js'),
child_process: '',
}
}
});
3. 手動捆綁模塊
如果您確實需要使用特定的 CommonJS 模塊,但又不希望啟用整個 Node.js API 的 polyfill,您可以在自己的腳本中捆綁這些模塊,然後像這樣在您的 Vue 組件中引入:
// 在 Vue3 + Vite 專案的文件中
import bundle from './bundle-third-party.js';
const MyThirdPartyModule = bundle(); // 呼叫捆綁腳本的回呼函數
console.log('MyThirdPartyModule:', MyThirdPartyModule);
捆綁腳本示例:
// bundle-third-party.js
const path = require('path');
const fs = require('fs');
const mkdirp = require('mkdirp');
const browserify = require('browserify');
const babel = require('rollup-plugin-babel');
function createBundle(modName, outputPath) {
return new Promise((resolve, reject) => {
let bundler;
if (outputPath && typeof outputPath === 'object') {
outputPath = outputPath[0];
}
if (!outputPath) {
outputPath = modName;
} else if (typeof outputPath === 'undefined') {
reject(`Output path must be a string or an array of strings. Got ${outputPath}`);
}
try {
bundler = browserify({
standalone: modName,
basedir: __dirname,
cache: {},
packageCache: {},
fullPaths: true,
entries: [`${__dirname}/${modName}`]
});
} catch (e) {
reject(e);
}
bundler.plugin(babel.default, {
exclude: ['node_modules/**'],
presets: [['@babel/env', { modules: false }]],
babelrc: false,
compact: false
});
mkdirp(path.dirname(outputPath));
bundler.bundle((err, src) => {
if (err) {
reject(err);
} else {
fs.writeFileSync(outputPath, src);
resolve();
}
});
});
}
module.exports = function() {
return createBundle('my-third-party-module', './dist/my-third-party-module.umd.js');
};
4. 使用 Webpack 作為外部工具
由於 Vite 本身不支持 CommonJS,有些情況下,使用 Webpack 作爲外部的打包工具可能是必要的。你可以根據自己的需求,選擇性地使用 Webpack 來處理某些特定場景下的打包任務。
總之,在使用 Vue3 + Vite 開發過程中,避免使用 `require` 是一個好習慣,特別是在客戶端代碼中。如果真的需要在瀏覽器環境中使用 Node.js 模塊,則需要適當地配置 polyfills 或使用其他方式來解決相容性的問題。