在現代網頁應用程式開發中,Vue.js 是一個極為流行的框架,其靈活且易於學習的特性備受開發者們喜愛。其中一個關鍵功能就是它的組件系統,這使得重用UI元素並將它們連接在一起變得非常簡單。然而,隨著應用程式的規模擴大,不同層級或不同區域之間的組件溝通需求也隨之增加。本篇文章將深入探討 Vue.js 中各種不同的組件間通信模式,幫助讀者理解並運用在實際項目中。
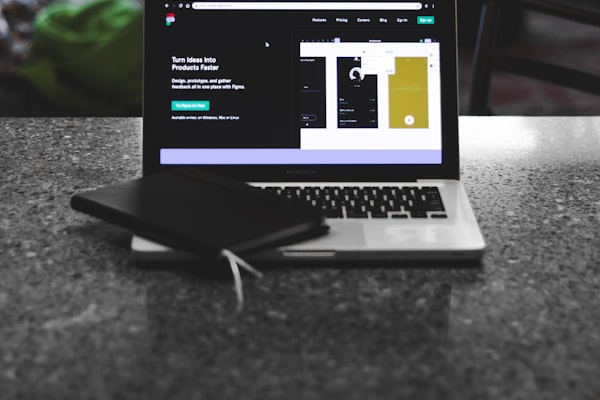
1. Props 和 Event Emit
這是 Vue.js 中一種最基礎也是最常用的組件溝通方式。父組件可以透過定義 props 來向子組件傳遞資料,而子組件則可以使用 `$emit` 方法來發送事件通知父組件更新某些狀態或者觸發某個動作。例如:
<!-- Parent Component (App.vue) -->
<template>
<div id="app">
<ChildComponent :message="greeting" @updateMessage="handleUpdateMessage" />
</div>
</template>
<script>
export default {
data() {
return {
greeting: 'Hello, World!'
};
},
methods: {
handleUpdateMessage(newGreeting) {
this.greeting = newGreeting;
}
}
};
</script>
<!-- Child Component (ChildComponent.vue) -->
<template>
<button @click="sendUpdatedMessage">Change Greeting</button>
</template>
<script>
export default {
props: ['message'], // 從父組件接收 message prop
methods: {
sendUpdatedMessage() {
// 使用 $emit 來發出 updateMessage 事件,帶有新的訊息字串作為參數
this.$emit('updateMessage', 'Bye-bye, World!');
}
}
};
</script>
在上面的例子中,當點擊「Change Greeting」按鈕時,ChildComponent 會發出一種名為 `updateMessage` 的事件,該事件會被 App.vue 的 `@updateMessage` 監聽器所捕獲到,進而觸發 `handleUpdateMessage` 函數來更新 greeting 屬性。這種方式適合於單向資料流,即資料只從父組件流向子組件,並且子組件能夠回調以通知父組件發生了變化。
2. Provide / Inject API
這個機制允許在任何地方共享一個依賴項(對象),而不需要基於繼承或硬編碼的依賴關係。它通常用於跨多個層次的組件之間進行通信,比如當您想要在某個高階範圍內共享單例服務時。以下是使用 `provide/inject` 的示例:
// 在根組件或在所有組件都共用的頂層範圍中提供依賴項
const provider = {
someService: new SomeService()
};
new Vue({
el: '#app',
provide: provider,
components: { /* ... */ }
});
然後在其他任何地方都可以注入這些依賴項:
// inject someService 到任何想要使用的組件中
export default {
inject: ['someService'], // 請注意這裡沒有 Provider 或 ProvidedIn 定義
created () {
console.log(this.someService); // 這裡可以存取到被提供的 service
}
}
3. 插槽 Slot
插槽是一種在組件內部傳遞內容的方式。它不僅可以用來實現模板的可重複利用,還可以在子組件與其容器父組件之間建立動態的內容交互。例如:
<!-- Parent Component -->
<template>
<MyCustomButton>
<!-- 此處的內容將會被渲染到 <slot/> 上 -->
Default text content of the button
</MyCustomButton>
</template>
<!-- MyCustomButton Component -->
<template>
<button class="custom-btn">
<slot></slot>
</button>
</template>
在上述情況下,Parent Component 中的文字 “Default text content of the button” 將會顯示在 MyCustomButton 裡的 “ 位置上。
4. 綁定屬性 Binding Properties
在一些較為複雜的情況下,你可能需要在不同的 Vuex 命名空間或不同層級的組件之間傳遞資料。這時候你可以考慮使用 `v-bind` 指令搭配特殊的屬性名稱來達成目的。以下是如何實作的一個簡化版示例:
// 在任何地方定義和使用
Vue.component('my-special-comp', {
name: 'mySpecialComp',
props: [ 'msg' ],
mounted () {
console.log(this.msg); // 輸出 'This is a special component'
}
})
// 在其他組件中這樣使用
<my-special-comp msg="This is a special component" />
在此案例中,我們透過 `msg` 屬性的值來向 `my-special-comp` 組件傳遞資訊。這個技巧可以視為是 props 和 event emit 的結合體,但它是直接通過 HTML 標記而不是事件來完成的。
5. Vuex
如果您的應用程序已經使用了 Vuex,那麼它可能是處理組件之間狀態管理的最合適解決方案。Vuex 提供了一個集中式的地方來存放所有的應用程式狀態,並且提供了嚴格的管理規則來確保狀態的一致性和可預測性。以下是如何使用 Vuex 來完成組件間通信的示例:
// store/index.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export const store = new Vuex.Store({
state: {
counter: 0
},
mutations: {
incrementCounter (state) {
state.counter += 1;
}
}
});
// Anywhere in your components
computed: {
currentCount () {
return this.$store.state.counter;
}
},
methods: {
incrementHandler () {
this.$store.commit('incrementCounter');
}
}
在這個例子中,任何擁有 `$store` 引用的組件都可以存取 `counter` 狀態並執行相應的 mutation 來改變它。這樣做的好處是保持了狀態的唯一真相源頭,並且讓狀態變化的歷史可以被追蹤和復原。
小結
Vue.js 提供了許多強大的工具來幫助開發人員解決組件之間的通信問題。選擇哪種方式取決於你的應用程序的需求以及各個組件之間的關係。瞭解每種模式的優缺點,將使你在設計和構建大型 Web 應用程序時更加遊刃有餘。