在Vue.js 3 中,使用純前端技術來導入Excel文件並將其中的數據解析出來,最終渲染到表格中,通常需要藉助第三方插件或者庫來實現這一功能。目前有許多這樣的插件可用,例如 `@excel-export/core` 和 `xlsx` 等。以下是如何使用這些插件的一個示例教程,請注意,由於篇幅限制,本文不會包含所有可能出現的錯誤處理或異常情況。
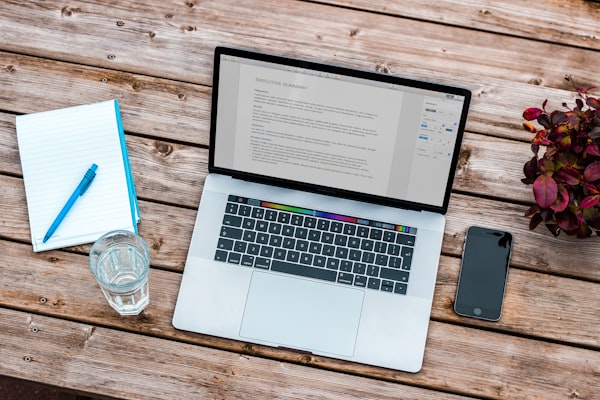
安裝必要的前端依賴包
首先,我們需要通過npm安裝兩個主要的依賴包:`@excel-export/core` 和 `filepond`。這兩個包分別用於讀取Excel文件內容以及上傳文件。
npm install @excel-export/core filepond --save
然後,確保在你的HTML頁面中正確地引入了以下腳本和樣式表:
<link href="https://unpkg.com/filepond/dist/filepond.css" rel="stylesheet">
<script src="https://unpkg.com/filepond/dist/filepond.js"></script>
<script src="node_modules/@excel-export/core/lib/cjs/index.umd.min.js"></script>
Vue組件的創建
接下來,我們將在Vue項目中創建一個新的組件,這個組件將負責從用戶那裏獲取Excel文件,解析其中的數據,並將結果顯示在一個表格中。我們將這個組件命名爲`ExcelImporter`。
ExcelImporter組件結構
下面是我們的組件的基本結構,它包含了FilePond的上傳組件和一個空的表格作爲數據的展示區域:
<template>
<!-- File Pond for uploading the excel file -->
<div class="filepond--wrapper">
<label for="excel-upload">選擇一個Excel文件</label>
<input id="excel-upload" type="file" name="files[]" multiple />
<FilePond ref="pond" :allowMultiple="false"/>
</div>
<!-- Table to display parsed data -->
<table>
<tr>
<th v-for="header in headers" :key="header">{{ header }}</th>
</tr>
<tr v-if="data.length > 0" v-for="row of data" :key="row.id">
<td v-for="(cell, index) in row" :key="index">{{ cell }}</td>
</tr>
<tr v-else>
<td>暫無數據</td>
</tr>
</table>
</template>
<script lang="ts" setup>
import { defineProps } from 'vue';
import FilePond from 'filepond';
const props = defineProps({}); // Your component's prop types go here
// The following code is written in TypeScript and assumes you are using TS with your Vue project
const handleFilesAdded = (files: any): void => {
console.log('Files added:', files);
};
// This function parses an Excel file using xlsx.full.min.js
async function parseExcel(blob: Blob): Promise<void> {
try {
await import('xlsx');
const workbook = XLSX.read(window.URL.createObjectURL(blob));
const firstSheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[firstSheetName];
// Parse the sheet into a JSON array
const rows = XLSX.utils.sheet_to_json(worksheet, { raw: true });
// Remove empty strings at beginning and end
rows.shift();
rows.pop();
// Store the column headers and the processed data
const headers = Object.keys(rows[0]);
const data = rows.slice(1);
// Update state
updateState({ headers, data });
} catch (error) {
console.error('Error parsing Excel file:', error);
}
};
function updateState(newData: { headers: string[]; data: object[] }): void {
console.log(`New data received:`, newData);
}
// Attach event listeners to FilePond instance
const filePondRef = ref<any>(null);
onMounted(() => {
filePondRef.value?.setOptions({
onaddfile: handleFilesAdded,
});
});
return {
headers: [],
data: [],
parseExcel,
filePondRef,
};
</script>
在這個組件中,`handleFilesAdded`函數會在用戶選擇了一個新的Excel文件後觸發,而`parseExcel`函數則會被用來實際執行對文件的解析操作。一旦數據被成功解析,就會更新`headers`和`data`的狀態,從而使得表格的內容得以更新。
在主App中使用ExcelImporter組件
最後一步是將`ExcelImporter`組件添加到你項目的根組件(通常是`App.vue`)中。確保你已經導入了該組件並在模板中正確地使用了它。
<template>
<div id="app">
<!-- Include your other components or content here -->
<ExceImporter />
</div>
</template>
<script lang="ts">
import ExceImporter from '@/components/ExceImporter.vue';
export default {
name: 'App',
components: {
ExceImporter,
},
};
</script>
現在,當你運行項目時,應該能夠在頁面上看到一個可以上傳Excel文件的區域,並且當文件被解析完成後,數據將會顯示在一個表格中。請記住,上述代碼只是一個起點,你可能需要在生產環境中進行更多的優化和安全措施。此外,在實際應用中,你可能還需要考慮不同瀏覽器對於JavaScript API的支持程度差異。